Python uses the time.sleep()
function to pause program execution for the defined time. Pausing code for a specified time is useful when a program requires delays.
The function also helps to prevent overloading a system with requests and introduces system delays when they are welcome.
This guide shows how to use time.sleep()
in Python. We will also cover its limitations and alternatives for different situations.
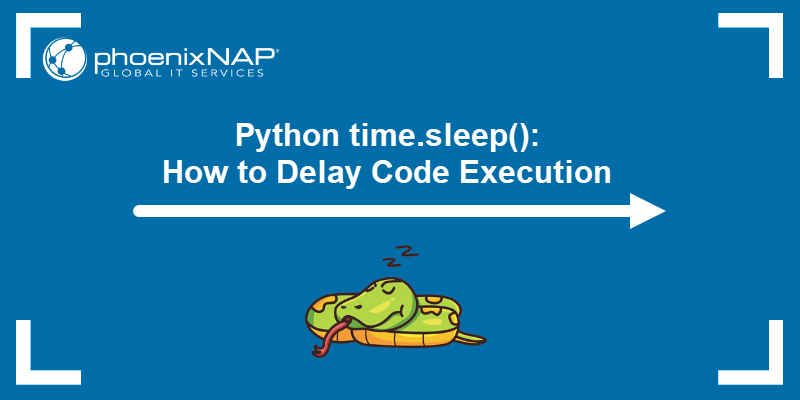
Prerequisites
- Python 3 installed (we're using Python 3.12).
- Access to a Python IDE or editor.
- A way to run the code (CLI or IDE).
Note: To install Python, follow one of our guides for your OS:
Python time.sleep() Syntax
The general syntax for the time.sleep()
function is:
import time
time.sleep(seconds)
The first line imports the time
library, while the second uses the method. Provide the time in seconds as an argument. The number can be an integer or a float for sub-second precision.
Note: Integer and float are both numeric data types. For more info, see our in-depth article about Python data types.
When to Use time.sleep() in Python?
There are many contexts where time.sleep()
is helpful. Below are some common scenarios where pausing code is necessary or required.
Threading
In a multi-threaded application, multiple threads can compete for limited system resources. Adding a time.sleep()
function between thread actions helps space out the execution. It allows threads to perform their task and reduces the risk of CPU overload.
For example, a worker thread that checks for new data can be paused for a few seconds between checks.
Web Scraping
Web scraping often requires making many requests to a website. Websites implement rate-limiting to prevent abuse; too many requests can result in an IP block.
To prevent this, developers add time.sleep()
between HTTP requests to control the request rate. It can simulate human-like behavior by introducing a wait of 1-2 seconds between actions to stay within acceptable limits.
Testing Delays
Different software testing methodologies, such as unit or integration testing, require simulating real-world conditions. To create an artificial processing delay, the time.sleep()
function can help mimic realistic network latency.
API Requests
Similar to web scraping, third-party API services also implement rate limits. For example, there might be a limit to how many requests can be made per minute. Failing to respect these limits results in slower responses over time or bans.
Adding time.sleep()
between requests helps control the number of calls and respects usage policies.
Loading Simulation
Command-line tools, user interfaces, and games use loading to improve UX. It makes room for animations, progress bars, or loading messages.
Loading simulations are easy to create with time.sleep()
and help prevent abrupt change. This action provides an opportunity for a more organic transition.
Hardware and Sensor Synchronization
A small wait often prevents communication errors in hardware components. A program that interacts with hardware devices (such as sensors or serial ports in a Raspberry Pi) uses time.sleep()
for synchronization. It helps pause a program until a device is ready or a measurement is complete.
time.sleep() Limitations
The time.sleep()
function is a simple way to pause a program. However, there are several drawbacks and limitations that make it unsuitable in some scenarios:
- Blocking behavior. The
time.sleep()
function blocks an entire thread for the provided duration. It halts code execution and prevents other tasks from being performed. It's unsuitable for parallel workloads and multithreading where blocking behavior is unwelcome. - Inaccurate. The sleep duration is not exact due to OS scheduling and context switching. The delay itself can be longer than expected. Real-time systems and time-critical applications require a more reliable timing mechanism.
- Hard to interrupt. Once a program is sleeping, a clean interrupt is hard to achieve.
Programs that require concurrency, precision, and responsiveness should use a different program pausing method.
Python time.sleep() Examples
This section shows several examples to demonstrate how time.sleep()
works. Use an IDE or code editor to write the code and test its functionality.
Basic Delay
The example below shows how to delay code using time.sleep()
:
import time
print("Code pausing for 5 seconds...")
time.sleep(5)
print("Done!")
The code prints a message, waits for 5 seconds, then prints another message once the time is up.
Delay in a Loop
Use time.sleep()
in a loop to delay each loop step.
For example:
import time
for i in range(3):
print("Step", i+1)
time.sleep(1)
The code prints the current step and waits 1 second before printing the following.
Float Delay
Use floating-point values to delay the program in milliseconds:
import time
print("Code pausing for 500 milliseconds...")
time.sleep(0.5)
print("Done!")
The code waits for half a second before printing the second message.
Python time.sleep() Alternatives
For better flexibility, accuracy, or non-blocking behavior, there are time.sleep()
alternatives. The sections below show these alternatives and explain when to use them instead.
threading.Event.wait()
Since time.sleep()
always blocks for a fixed duration, it's unsuitable for coordinating threads or allowing interrupts. The threading.Event.wait()
function is better when a thread needs to wait for a condition or timeout, but still be responsive.
For example:
from threading import Event
event = Event()
print("Waiting 5s...")
event.wait(5)
print("Done.")
The code waits for 5 seconds. However, in another thread, the wait can be interrupted early with event.set()
.
For example:
from threading import Event, Thread
import time
event = Event()
def trigger_event():
time.sleep(1)
event.set()
Thread(target=trigger_event).start()
print("Waiting...")
event.wait(5)
print("Done. Waited for 1 second instead of 5 seconds.")
Use the function when coordinating threads and when requiring interruptible sleep.
threading.Timer()
To delay code without interrupting the current thread, use the threading.Timer()
function. The method runs a task after a specified delay in a new thread.
The following example shows how to schedule a function to run in 60 seconds in another thread:
import threading
def my_function():
print("This one after 5 seconds.")
threading.Timer(5, my_function).start()
print("This line executes first.")
Use this method to defer the execution of some task to another thread without blocking your application.
asyncio.sleep()
For asynchronous (async/await) code, use the asynchio.sleep()
function. It allows other async tasks to run while it waits.
For example:
import asyncio
async def start_end():
print("Start")
await asyncio.sleep(5)
print("End")
async def middle():
await asyncio.sleep(2.5)
print("Middle")
async def main():
await asyncio.gather(start_end(), middle())
asyncio.run(main())
The function is commonly used for web servers, applications with many I/O tasks, and chatbots.
Sleep in Different Python Libraries
Many Python third-party libraries have their own version of sleep to support different uses. These functions are often tailored to work better within their framework and for better integration.
Some examples are shown in the sections below. The libraries in these sections are non-standard and require installation using a package manager (such as PIP).
Note: To install PIP, follow one of our guides:
gevent
The gevent library is used in concurrent programming to provide a greenlet-based coroutine model.
Note: Greenlets are lightweight pseudo-threads.
The library replaces Python's standard time.sleep()
function with a non-blocking alternative to simplify concurrency.
The example below shows how the sleep function works in gevent:
import gevent
from gevent import monkey
monkey.patch_all()
def task(name):
print(f"{name} starting.")
gevent.sleep(5)
print(f"{name} finished.")
gevent.joinall([
gevent.spawn(task, 'First task'),
gevent.spawn(task, 'Second task'),
])
The sleep function is non-blocking and yields to other greenlets. It's ideal for scalable I/O-bound applications.
twisted
Twisted is a framework for network programming written in Python. It's used in event-driven programming, protocols, and services.
To schedule delays and callbacks, it uses a reactor.callLater()
method instead of time.sleep()
:
from twisted.internet import reactor
def delayed_task():
print("This runs after a 5 seconds.")
reactor.stop()
reactor.callLater(5, delayed_task)
print("Reactor running...")
reactor.run()
The function is non-blocking and works as a scheduling mechanism.
Conclusion
This guide showed how to use the time.sleep()
Python function to delay code execution through examples. It also explained when to use it and alternatives for different situations.
Next, see how to get started with web application development and install Flask.