A program is a structured set of instructions designed to perform specific tasks or solve problems. It can range from simple scripts to complex applications, serving various purposes in areas such as education, business, and entertainment.
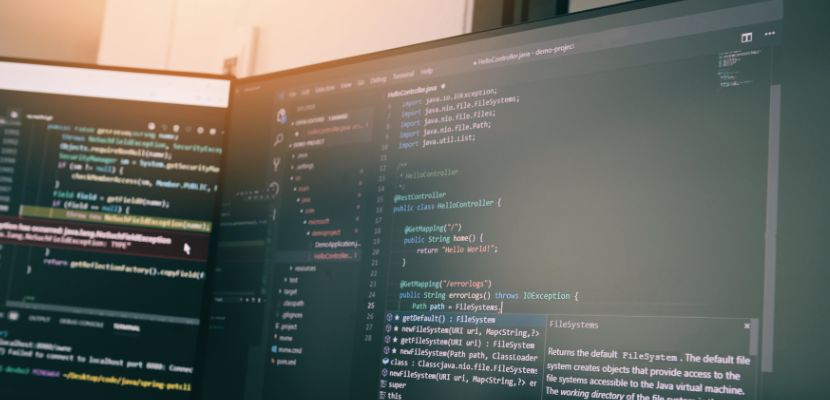
What Is the Definition of a Program?
A program is a cohesive sequence of instructions written in a programming language, designed to perform a specific function or set of functions when executed by a computer or other digital device. It operates by processing inputs, executing logical and mathematical operations, and generating outputs or performing actions based on predefined rules.
Programs are built to automate tasks, solve problems, or manage data, often functioning as the core component of applications, systems, or devices. They encompass algorithms, logic, and control structures that define how information is handled, ensuring the desired outcomes are achieved efficiently and accurately. Programs are integral to modern technology, enabling everything from basic computations to complex systems like artificial intelligence and enterprise software.
Modes of a Program
Programs often operate in different modes to provide flexibility and functionality tailored to specific tasks or environments. These modes determine how the program interacts with the user, processes data, or behaves in varying conditions. Here are some common modes of operation.
Interactive Mode
Interactive mode allows a program to directly engage with users by processing inputs in real time and providing immediate feedback. This mode is common in applications like command-line interfaces or software tools where users enter commands or data, and the program responds dynamically. Interactive mode is ideal for tasks requiring frequent user input or decision-making, offering a highly responsive and user-driven experience.
Batch Mode
Batch mode processes a predefined set of tasks or data without requiring user interaction during execution. Typically used for automating repetitive processes, this mode is efficient for scenarios like file conversions, large-scale data analysis, or system updates. Programs operating in batch mode execute commands sequentially, often relying on scripts or scheduled tasks, making it suitable for operations that can run unattended.
Daemon or Background Mode
Daemon or background mode is designed for programs that run continuously in the background, often without direct user interaction. Common in system utilities and server processes, this mode ensures the program is always available to perform tasks such as monitoring, logging, or responding to system events. Background mode is crucial for maintaining system stability and handling tasks that require ongoing availability.
Safe Mode
Safe mode is a diagnostic or troubleshooting mode that runs a program or operating system with minimal functionality, bypassing non-essential components. It is often used to identify and resolve issues caused by faulty configurations, software conflicts, or hardware failures. By operating in a controlled environment, safe mode helps users and technicians isolate and fix problems without interference from additional features.
Simulation Mode
Simulation mode replicates the behavior of a program or system under controlled conditions to test or predict outcomes without affecting real-world operations. It is widely used in training, research, and development to model scenarios, analyze potential risks, or refine processes. Simulation mode enables experimentation and learning, often incorporating detailed data and realistic variables to enhance its accuracy.
What Are the Components of a Program?
The components of a program are the fundamental building blocks that define its structure, functionality, and behavior. Together, these components enable a program to execute specific tasks and deliver desired outcomes. Key components include:
- Code. The code is the core of any program, consisting of instructions written in a programming language. It defines the logic, algorithms, and control flow that dictate how the program operates. Code is structured into functions, methods, or procedures, each handling specific tasks or operations.
- Input. Input represents the data or commands provided to a program by users, sensors, or other systems. This data serves as the starting point for processing and can come from various sources, such as keyboards, files, or network connections. Input is crucial for dynamic and interactive functionality.
- Output. Output is the result produced by the program after processing input or executing its logic. It can take various forms, including text on a screen, generated files, or signals sent to other devices. Output is the program's way of communicating its results or actions to users or external systems.
- Data structures. Data structures are organizational methods used to store and manage data within a program. They include arrays, lists, dictionaries, and more complex structures like trees and graphs. These structures enable efficient data manipulation, retrieval, and storage.
- Control structures. Control structures guide the flow of a program's execution. They include loops, conditionals, and branching statements that determine how and when specific parts of the program are executed. Control structures allow for dynamic decision-making and iterative processes.
- Error handling. Error handling ensures that a program can detect, respond to, and recover from unexpected issues or exceptions during execution. By implementing mechanisms like try-catch blocks, error messages, and fallback routines, programs maintain stability and reliability even when problems arise.
- Documentation. Documentation includes comments within the code and external files that explain the program's purpose, structure, and functionality. It aids developers in understanding and maintaining the program, ensuring consistency and facilitating collaboration.
What Is a Program Example?
Microsoft Word is a widely recognized program designed for word processing. It allows users to create, edit, and format text documents, offering features such as spell check, text styling, tables, and templates. Behind the scenes, Microsoft Word operates through a combination of code, data structures, and user interfaces, processing inputs (like text typed on the keyboard) and generating outputs (formatted documents).
This example illustrates how a program serves a practical function, leveraging computational processes to enhance productivity and address user needs.
What Is a Program Used For?
A program is used to execute specific tasks, solve problems, or automate processes across a wide range of applications. Programs are designed to process data, perform calculations, manage systems, or facilitate user interactions, making them essential tools in virtually every field.
For example, programs can be used for word processing, managing databases, analyzing data, controlling machinery, or creating and editing media. In business, they streamline operations such as accounting, customer relationship management, and inventory tracking. In science and research, programs enable simulations, modeling, and the analysis of complex datasets. For personal use, programs power applications like social media platforms, video games, and productivity tools.
Who Creates a Program?
A program is created by software developers or programmers, professionals who write and design the code that forms the foundation of the program. These individuals use programming languages, tools, and frameworks to develop software tailored to specific tasks or requirements.
Program creation often involves a collaborative effort within a development team, including roles such as:
- Software engineers who design the overall system architecture and ensure the program's functionality aligns with user needs.
- Frontend developers who focus on creating the user interface and user experience (UI/UX) aspects of the program.
- Backend developers who handle server-side logic, databases, and the integration of the program with other systems.
- Quality Assurance (QA) testers who test the program for bugs and ensure it meets performance and usability standards.
Can You Create Your Own Program?
Yes, you can create your own program, even if you're new to programming. With the right tools, knowledge, and resources, anyone can develop a program tailored to specific needs or goals. Hereโs how you can get started:
- Identify the purpose. Decide what you want your program to do. Whether it's a calculator, a simple game, or a tool to automate tasks, having a clear objective is crucial.
- Learn a programming language. Choose a language suited to your goals. For beginners, Python is often recommended due to its simplicity and versatility. Other popular languages include JavaScript for web development or Java for broader applications.
- Use development tools. Install an integrated development environment (IDE) like Visual Studio Code, PyCharm, or Eclipse, which provides a user-friendly interface for writing and testing code.
- Write the code. Start small by creating basic programs to understand the syntax and logic of your chosen language. Gradually, you can work on more complex projects.
- Test and debug. Run your program to identify errors (bugs) and fix them. Testing ensures your program performs as intended under various conditions.
- Iterate and improve. Enhance your program by adding features, optimizing performance, or improving the user interface.
How Is a Program Created?
Creating a program involves a structured process that transforms an idea into a functional software application. Hereโs an overview of the key steps involved in program creation:
- Define the purpose and requirements. The first step is identifying the problem the program will solve or the task it will perform. Clear requirements are gathered, outlining the program's goals, features, and functionality. This step ensures alignment with user needs or project objectives.
- Design the architecture. A plan or blueprint is created to outline how the program will work. This includes designing the system architecture, determining the flow of data, and defining how different components will interact. Tools like flowcharts, wireframes, or UML diagrams are often used.
- Write the code. Using a programming language suited to the task, developers write the code that implements the programโs logic. This involves creating algorithms, structuring data, and building the user interface. Developers may use an integrated development environment to streamline coding.
- Test the program. After writing the initial code, the program undergoes rigorous testing to identify and fix bugs, performance issues, or usability problems. Testing can include unit tests (individual components), integration tests (interaction between components), and user acceptance tests.
- Debug and refine. Errors or inefficiencies discovered during testing are resolved by debugging. Developers may also optimize the programโs performance, improve the user interface, or add enhancements based on feedback.
- Deploy the program. Once the program is complete and meets all requirements, it is deployed to its intended environment. This could involve installing the program on a computer, publishing it to an app store, or deploying it to a server for web or cloud-based applications.
- Maintain and update. After deployment, the program is monitored for issues, updated to add features or improve functionality, and maintained to ensure compatibility with new systems or technologies.
How Long Does It Take to Create a Program?
The time it takes to create a program varies widely and depends on several factors, including the program's complexity, scope, purpose, and the resources available. Below are some key considerations that influence the timeline:
- Complexity and size. A simple program, such as a basic calculator or a script to automate tasks, can be created in a few hours or days. However, larger, more complex programsโlike enterprise applications, video games, or operating systemsโtake months or even years to develop.
- Team size and expertise. A small team or a solo developer may require more time compared to a larger team with specialized roles. The expertise of the developers also plays a significant role; experienced programmers often work faster and more efficiently.
- Development methodology. The chosen development methodology affects the timeline. Agile methods, for example, prioritize iterative progress with regular updates, allowing parts of the program to be delivered quickly. Traditional waterfall methods may take longer as they focus on completing each phase before moving to the next.
- Tools and frameworks. Modern development tools, libraries, and frameworks significantly speed up the process by providing prebuilt components, templates, or automation for repetitive tasks.
- Testing and debugging. Thorough testing and debugging are critical for a programโs success. Simple programs may only require minimal testing, while complex systems often undergo extended testing cycles to ensure reliability.
- Maintenance and updates. A program is rarely "finished" after its initial release. Time must also be allocated for future updates, feature additions, and compatibility improvements, which extend the overall lifecycle of development.
Are Programs Free?
Programs can be either free or paid, depending on their purpose, development model, and licensing terms. Here's an overview of the different types:
- Free programs. Many programs are free to use and are often referred to as freeware or open-source software. Freeware programs, such as popular web browsers or basic utility tools, are provided at no cost but may have limitations or advertisements. Open-source programs, like the Linux operating system, are not only free but also allow users to modify and distribute the source code under certain licenses, such as the GPL (General Public License).
- Paid programs. Other programs require payment, either as a one-time purchase or through subscription models. Paid software often provides advanced features, customer support, and regular updates. Examples include professional tools like Adobe Photoshop or enterprise-grade software like Microsoft Office 365.
- Freemium programs. Some programs follow a freemium model, offering basic features for free while charging for premium features or additional services. For instance, many mobile apps provide free access to core functionality but require in-app purchases or subscriptions for advanced tools.
- Trial or demo programs. Certain paid programs offer free trials or demo versions, allowing users to test the software before committing to a purchase. These versions are often time-limited or feature-restricted.
What Is the Difference Between a Program and a Script?
Hereโs a table comparing the differences between a program and a script:
Aspect | Program | Script |
Definition | A standalone software application designed to perform a wide range of tasks. | A smaller, lightweight code designed to automate specific tasks. |
Complexity | Typically more complex, with extensive features and functionality. | Generally simpler and focused on a specific task or workflow. |
Execution | Requires compilation (for compiled languages) or execution via runtime environments. | Runs directly in an interpreter, such as Python, Bash, or JavaScript. |
Size | Often larger and includes multiple files, libraries, and modules. | Usually concise and contained in a single file. |
Development time | Takes longer to develop due to complexity and feature scope. | Faster to create and deploy for quick automation or prototyping. |
Use case | Designed for robust, scalable, and long-term solutions. | Used for automating repetitive tasks, testing, or small-scale operations. |
Examples | Microsoft Word, web browsers, enterprise software. | Shell scripts, Python scripts for data processing, JavaScript for webpages. |
Portability | May require specific platforms or environments to run. | Often highly portable and runs on multiple platforms with minimal setup. |
Maintenance | Requires structured updates, testing, and long-term support. | Easier to modify and maintain due to its simplicity. |