JavaScript is a versatile programming language commonly used to create interactive effects within web browsers. As a core technology of the World Wide Web, alongside HTML and CSS, JavaScript enables dynamic content, control of multimedia, and animation on web pages.
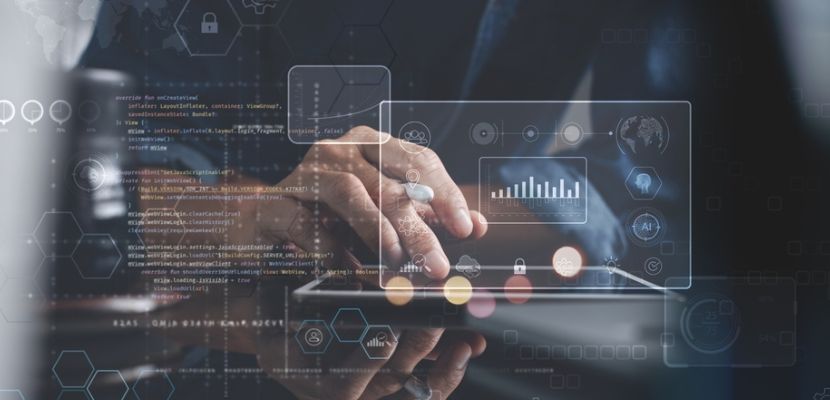
What Is JavaScript?
JavaScript is a high-level, interpreted programming language that is primarily known for its role in enhancing web pages to provide a more dynamic and interactive user experience. Developed initially by Netscape, JavaScript has evolved to become one of the core technologies of the World Wide Web, alongside HTML and CSS.
JavaScript is an object-oriented language, allowing developers to create complex features on web pages, such as real-time content updates, interactive forms, animations, and multimedia handling. It runs on the client side, meaning it is executed by the user's web browser, which reduces the load on web servers and can result in faster page interactions. The language is known for its event-driven, functional, and imperative programming styles, making it flexible and adaptable to various development needs.
How Does JavaScript Work?
JavaScript executes code within a web browser, enabling dynamic interactions and functionalities on web pages. Here’s a detailed explanation of how JavaScript operates:
- Integration with HTML and CSS. JavaScript is typically embedded within HTML documents. It can be included directly in HTML files using <script> tags or referenced externally via linked script files. It also interacts with CSS to dynamically modify the appearance of web pages.
- Execution environment. JavaScript code is executed by the browser’s JavaScript engine, which interprets and runs the script. Popular JavaScript engines include Google’s V8 (used in Chrome and Node.js), Mozilla’s SpiderMonkey (used in Firefox), and Microsoft’s Chakra (used in Edge).
- Event-driven programming. JavaScript is event-driven, meaning it responds to user interactions such as clicks, keypresses, and mouse movements. Developers can define event handlers—functions that execute in response to specific events—to create interactive features on web pages.
- Document object model (DOM) interaction. JavaScript can access and manipulate the DOM, a tree-like structure representing the HTML elements of a web page. By interacting with the DOM, JavaScript can dynamically update content, styles, and structure without requiring a page reload. For instance, it can add new elements, modify existing ones, or remove elements from the page.
- Asynchronous operations. JavaScript supports asynchronous programming, allowing operations like fetching data from a server to run in the background without blocking the main execution thread. This is achieved through callbacks, promises, and the async/await syntax. Asynchronous operations are essential for creating smooth and responsive user experiences.
- Variables and data types. JavaScript uses variables to store data. Variables hold different data types, including numbers, strings, objects, arrays, and functions. The language is dynamically typed, meaning variables do not need a defined data type and can change types at runtime.
- Functions and scope. Functions are reusable blocks of code that can be defined and invoked to perform specific tasks. JavaScript supports various function types, including regular functions, arrow functions, and anonymous functions. Functions can also create local scopes, limiting the visibility of variables defined within them.
- Objects and prototypes. JavaScript is an object-oriented language, where objects are collections of properties and methods. Objects can be created using constructors or object literals. JavaScript uses prototypes for inheritance, allowing objects to share properties and methods through a prototype chain.
- Closures and higher-order functions. Closures are functions that retain access to their lexical scope, even when executed outside that scope. Higher-order functions are functions that take other functions as arguments or return them as results. These features enable powerful patterns for abstraction and code reuse.
- Error handling. JavaScript provides mechanisms for error handling through try, catch, and finally blocks. These constructs allow developers to manage exceptions and ensure the application can gracefully handle unexpected situations.
- Execution flow. JavaScript execution starts at the top of the script and proceeds sequentially. However, control structures like loops, conditionals, and function calls can alter this flow, allowing for complex logic and behaviors.
A Short History of JavaScript
JavaScript was created in 1995 by Brendan Eich while he was working at Netscape Communications Corporation. The language was initially developed in just ten days and was originally named Mocha, later renamed to LiveScript, and finally to JavaScript to capitalize on the popularity of Java at the time.
Released with Netscape Navigator 2.0 in 1995, JavaScript quickly became an essential tool for web developers due to its ability to create dynamic and interactive web pages. In 1996, Netscape submitted JavaScript to ECMA International for standardization, resulting in the ECMAScript specification. The first edition of ECMAScript was published in 1997.
Microsoft introduced its own version of JavaScript, called JScript, in Internet Explorer 3.0. This led to a period of browser incompatibility issues, which gradually improved as browsers adhered more closely to the ECMAScript standards.
Over the years, JavaScript has evolved significantly, with major updates like ECMAScript 5 (2009) introducing features such as strict mode, and ECMAScript 6 (2015), also known as ES6 or ECMAScript 2015, adding significant enhancements like classes, modules, and arrow functions.
Today, JavaScript is a cornerstone of web development, supported by all major browsers, and continues to evolve with annual updates to the ECMAScript specification. Its ecosystem includes numerous libraries and frameworks, making it one of the most versatile and widely used programming languages in the world.
JavaScript Use Cases
JavaScript is a versatile language with a wide range of use cases:
- Web development. One of its primary uses is enhancing web pages by enabling dynamic and interactive content. It is also used for form validation, providing immediate feedback to users without needing to reload the page. Another significant application is in creating single-page applications (SPAs) with frameworks like Angular, React, and Vue.js, which offer a seamless user experience by dynamically loading content.
- Server-side development. JavaScript is utilized in server-side development through Node.js, which allows developers to build scalable and high-performance network applications. In mobile app development, JavaScript frameworks such as React Native and Ionic enable the creation of cross-platform mobile applications from a single codebase.
- Game development. Game development is another area where JavaScript shines, with libraries like Phaser enabling the creation of browser-based games. Additionally, JavaScript is used in data visualization, leveraging libraries like D3.js to create interactive and visually appealing charts and graphs.
- Connected device management. With the advent of the Internet of Things (IoT), JavaScript has found use in controlling and managing connected devices. JavaScript is also integral to progressive web apps (PWAs), which combine the best features of web and mobile applications, offering offline capabilities and improved performance.
JavaScript Advantages and Disadvantages
When evaluating JavaScript as a programming language, it's essential to consider its advantages and disadvantages. Understanding these can help developers make informed decisions about when and how to use JavaScript effectively in their projects.
Advantages
JavaScript offers numerous advantages that make it a popular choice among developers for a wide range of applications. Understanding these advantages will provide insight into why JavaScript remains a cornerstone technology for building dynamic and responsive web experiences:
- Versatility. JavaScript can be used for both client-side and server-side development. This versatility allows developers to build entire applications using a single language, streamlining the development process and improving consistency across the codebase.
- Interactivity. JavaScript enables the creation of highly interactive web pages. It allows developers to implement features such as real-time updates, animations, and dynamic forms, enhancing user experience and engagement.
- Wide browser support. JavaScript is supported by all modern web browsers, ensuring that code written in JavaScript can run on virtually any device with a web browser. This broad compatibility makes it a reliable choice for web development.
- Rich ecosystem. JavaScript has a vast ecosystem of libraries and frameworks, such as React, Angular, and Vue.js, which help developers build complex applications more efficiently. Additionally, package managers like npm provide easy access to a wide range of reusable code modules.
- Community and resources. JavaScript has a large and active developer community, which means there are abundant resources for learning and problem-solving.
- Asynchronous programming. JavaScript supports asynchronous programming through callbacks, promises, and async/await. This allows developers to handle tasks like data fetching and event handling more efficiently, improving application performance and responsiveness.
- Prototypal inheritance. JavaScript's prototypal inheritance model provides flexibility in object-oriented programming. Developers can create objects and reuse code in a manner that suits their needs, promoting efficient code organization and maintenance.
- Integration capabilities. JavaScript easily integrates with other technologies and languages, such as HTML, CSS, and various backend languages. This interoperability makes it a valuable tool for full-stack development.
- Rapid development. With the help of modern frameworks and tools, JavaScript enables rapid development and prototyping. Developers can quickly build and test applications, making it an ideal choice for startups and agile development teams.
- Event-driven. JavaScript's event-driven nature allows for the creation of highly responsive applications which efficiently handle events such as user inputs, network responses, and time-based triggers.
Disadvantages
While JavaScript offers many advantages, it also comes with certain drawbacks. Understanding these disadvantages is crucial for making informed decisions about when and how to use JavaScript in your projects. This section will outline the key limitations and challenges associated with JavaScript, providing a balanced view of its potential pitfalls.
- Browser compatibility issues. Despite wide browser support, different browsers may interpret JavaScript code differently, leading to compatibility issues. Developers often need to write additional code or use polyfills to ensure consistent behavior across all browsers.
- Security vulnerabilities. JavaScript is executed on the client side, which can expose it to various security risks, such as cross-site scripting (XSS) attacks. Malicious code can exploit vulnerabilities, potentially compromising user data and application integrity.
- Performance limitations. JavaScript is an interpreted language, which can result in slower execution compared to compiled languages like C++ or Java. For performance-critical applications, this can be a significant drawback.
- Debugging complexity. Debugging JavaScript can be challenging, especially in large codebases or when dealing with asynchronous code. Although modern tools and browsers offer debugging capabilities, identifying and resolving issues can still be time-consuming and complex.
- Lack of static typing. JavaScript is dynamically typed, meaning variables can change types at runtime. While this provides flexibility, it can also lead to bugs that are difficult to detect and fix. Static typing, as found in languages like TypeScript, helps mitigate this issue, but it requires additional setup and learning.
- Inconsistent development practices. JavaScript’s flexibility allows for various coding styles and practices, which can lead to inconsistent code quality and maintainability. Adopting standardized coding guidelines and tools like linters can help, but they are not always enforced.
- Dependence on the client. As a client-side language, JavaScript relies on the user's device and browser to execute code. This dependence can lead to performance variability based on the user’s hardware and software environment, potentially affecting the user experience.
- Frequent updates and changes. The JavaScript ecosystem evolves rapidly, with frequent updates and new frameworks or libraries emerging. Keeping up with these changes can be challenging for developers, leading to issues with code deprecation and compatibility.
- Memory management. JavaScript's automatic memory management, while convenient, can sometimes lead to issues such as memory leaks. Developers need to be aware of how their code handles memory to avoid performance degradation over time.
- Complexity in large applications. As applications grow in size and complexity, managing JavaScript code can become difficult. Without proper architecture and organization, large codebases can become unwieldy and harder to maintain. Using patterns and frameworks can help, but they also add to the learning curve.
What Does JavaScript Do on a Web Page?
JavaScript enhances web pages by adding interactivity, dynamic content, and responsive user interfaces. Here are the key functions JavaScript performs on a web page:
- Manipulates HTML and CSS. JavaScript can change the structure and style of a web page by manipulating the document object model (DOM). It can add, remove, or modify HTML elements and adjust CSS styles dynamically, allowing for real-time updates without reloading the page.
- Handles events. JavaScript enables web pages to respond to user actions such as clicks, key presses, mouse movements, and form submissions. Event listeners can be attached to HTML elements to trigger specific functions when these actions occur, enhancing interactivity.
- Validates forms. JavaScript can validate user input in web forms before submission, ensuring data accuracy and completeness. This reduces server load by catching errors on the client side and providing immediate feedback to users.
- Fetches and sends data. Through technologies like AJAX (Asynchronous JavaScript and XML) and Fetch API, JavaScript communicates with web servers to fetch or send data asynchronously. This allows web pages to update content dynamically without a full page reload.
- Creates animations. JavaScript can animate HTML elements, creating smooth transitions and visual effects. It can move elements, fade them in or out, change colors, and more, enhancing the visual appeal of a web page.
- Manages cookies and local storage. JavaScript can read, write, and delete cookies, as well as use local and session storage to store data on the client side. This capability allows for the storage of user preferences, session data, and other information that enhances user experience.
- Builds single page applications (SPAs). With frameworks like React, Angular, and Vue.js, JavaScript can create SPAs that load a single HTML page and dynamically update the content as users interact with the app.
- Interacts with APIs. JavaScript can interact with various APIs (Application Programming Interfaces) to integrate external services and data into web pages. This includes third-party services like Google Maps, payment gateways, social media platforms, and more.
- Implements complex logic. JavaScript can handle complex programming logic to create sophisticated applications directly within the browser. This includes tasks such as data processing, user authentication, game development, and more.
- Supports asynchronous programming. JavaScript's support for asynchronous programming with callbacks, promises, and async/await enables the handling of concurrent operations efficiently. This is crucial for tasks like fetching data, handling user inputs, and performing background processing without blocking the main execution thread.