Perl is a versatile, high-level programming language known for its text processing capabilities and flexibility. Originally developed for report generation and scripting, it has evolved into a powerful tool for tasks ranging from system administration to web development.
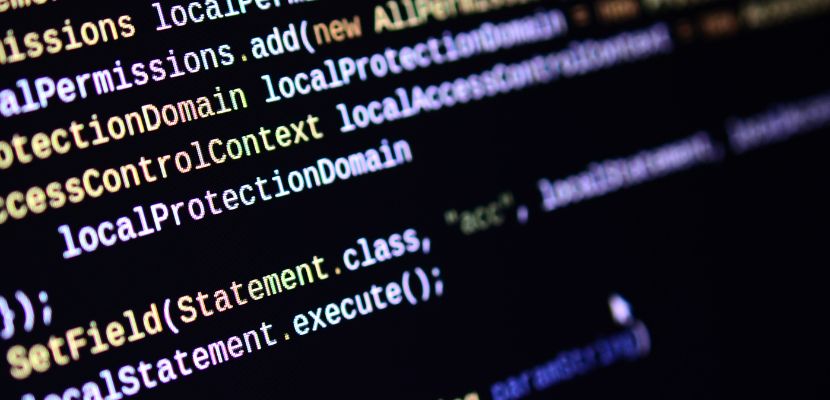
What Is Perl?
Perl is a high-level, general-purpose programming language that excels in tasks involving text manipulation and data parsing. Originally developed by Larry Wall in 1987, Perl was designed as a practical, efficient tool for scripting, automating processes, and generating reports. Its syntax combines elements from languages like C, sed, awk, and shell scripting, offering a balance of power and simplicity.
Over time, Perl has evolved into a dynamic language capable of handling a broad range of tasks, including system administration, web development, network programming, and database interaction. It is particularly valued for its ability to handle regular expressions and complex text processing with ease, making it a go-to language for tasks involving pattern matching and string manipulation. Perlโs flexibility, combined with its robust library ecosystem and cross-platform compatibility, allows developers to create efficient and scalable solutions across various domains.
Perl Syntax and Code
Perl syntax is a blend of simplicity and flexibility, drawing inspiration from languages like C, shell scripting, and sed. It provides multiple ways to accomplish the same task, allowing developers to choose the style that best suits their needs. Here's an overview of how Perl syntax and code work:
Variables and Data Types
Perl uses three primary types of variables, each distinguished by a sigil:
- Scalars ($). Hold single values like numbers or strings (e.g., $name = "Alice";).
- Arrays (@). Store ordered lists of scalars (e.g., @colors = ("red", "green", "blue");).
- Hashes (%). Store key-value pairs (e.g., %ages = ("Alice" => 30, "Bob" => 25);).
Operators
Perl supports a wide range of operators, including arithmetic (+, -, *), string concatenation (.), and comparison (==, eq). Special operators like =~ and !~ are used for regular expressions.
Control Structures
Perl offers common control structures such as if, unless, while, for, and foreach. These can be written in traditional block syntax or as statement modifiers for concise expressions (e.g., print "Hello" if $greet;).
Regular Expressions
One of Perlโs most powerful features is its support for regular expressions, used for pattern matching and text manipulation. Patterns are enclosed in / / and applied using the =~ operator (e.g., $text =~ /pattern/;).
Functions and Subroutines
Functions are built-in or user-defined and are invoked using their name followed by arguments in parentheses (optional in some cases). Subroutines are user-defined functions, declared with the sub keyword (e.g., sub greet { print "Hello"; }).
Context Sensitivity
Perl operates in scalar or list context, depending on how an expression is used. This context sensitivity allows the same expression to behave differently based on its surrounding code.
File and Input/Output
Perl provides powerful I/O capabilities, using functions like open, print, and readline. Filehandles are used to interact with files or streams, and Perlโs default variable $_ simplifies processing input line-by-line.
Comments and Documentation
Comments begin with # and extend to the end of the line. Documentation blocks are enclosed in =pod and =cut for longer descriptions.
Example Code
#!/usr/bin/perl
use strict;
use warnings;
my $name = "Alice"; # Scalar variable
my @colors = ("red", "blue"); # Array
my %ages = ("Alice" => 30); # Hash
print "Hello, $name!\n"; # Print with interpolation
foreach my $color (@colors) { # Loop through array
print "$color\n";
}
if ($ages{"Alice"} == 30) { # Hash lookup and conditional
print "Age is 30\n";
}
# Regular Expression
$name =~ s/Alice/Bob/; # Substitute "Alice" with "Bob"
print "New name: $name\n";
Perl Features
Perl is a highly versatile and dynamic programming language that blends simplicity with powerful tools for text processing, system automation, and beyond. Its rich feature set makes it suitable for a wide range of tasks, from small scripts to complex applications. Below are some of Perlโs defining features, explained in detail:
- Text processing and regular expressions. Perl is renowned for its text manipulation capabilities, especially through its built-in support for regular expressions. It allows complex pattern matching, substitution, and extraction with concise and expressive syntax. For example, finding and replacing text in a file or parsing structured data is straightforward with Perl.
- Dynamic typing and context sensitivity. Variables in Perl do not require explicit type declarations, making it dynamically typed. Additionally, Perl operates in scalar or list context based on how expressions are used, allowing the same code to behave differently depending on context. This flexibility simplifies coding but requires careful handling.
- Versatile data structures. Perl provides support for scalars, arrays, and hashes, which can represent everything from single values to complex data mappings. Nested and multidimensional data structures are also possible, making it easy to handle complex datasets.
- Cross-platform compatibility. Perl is highly portable and runs on a variety of platforms, including UNIX, Linux, Windows, and macOS. Code written in Perl can often be executed without modification across different operating systems.
- Comprehensive CPAN Library. The Comprehensive Perl Archive Network (CPAN) is a vast repository of pre-written modules and libraries. It covers nearly every conceivable task, from web development and database interaction to cryptography and bioinformatics, greatly accelerating development time.
- Powerful file and I/O handling. Perl simplifies interaction with files and streams through built-in functions like open, read, and print. Its default variable ($_) and line-by-line processing make scripting tasks like log parsing or file conversion intuitive and efficient.
- Flexibility and TMTOWTDI. Perl embodies the philosophy "There's More Than One Way To Do It" (TMTOWTDI), encouraging developers to approach problems in various ways. This flexibility makes Perl adaptable to individual coding styles and problem requirements.
- Extensibility and embeddability. Perl can be extended with C/C++ code for performance-critical tasks and embedded into other programs, allowing it to be integrated into larger systems.
- Error handling and debugging. Perl offers robust error handling through modules like eval for trapping exceptions and built-in warnings and strict modes (use warnings; use strict;) to catch potential errors during development.
- Object-oriented programming support. While Perl is not an object-oriented language by default, it supports object-oriented programming through modules and built-in syntax. This allows developers to create reusable and modular codebases.
- Unicode and multilingual support. Perl provides comprehensive support for Unicode, making it suitable for applications requiring multilingual text processing or internationalization.
- Networking and web development. Perl is well-equipped for network programming and web development, with modules like LWP, HTTP::Request, and frameworks such as Catalyst and Mojolicious for creating dynamic web applications.
What Is Perl Used For?
Perl is a versatile and powerful programming language used across a wide range of domains due to its flexibility and strong text processing capabilities. Below are some of the primary applications of Perl:
1. Text Processing and Data Parsing
Perlโs strength in regular expressions makes it ideal for tasks involving text manipulation, such as:
- Searching and replacing text in large files.
- Parsing structured data formats like XML, CSV, and JSON.
- Cleaning and transforming datasets.
2. System Administration
Perl is a favorite among system administrators for automating repetitive tasks. Examples include:
- Writing scripts for file management and log analysis.
- Monitoring system performance and generating reports.
- Managing network configurations and user accounts.
3. Web Development
Perl played a significant role in early web development and continues to be used for:
- Building dynamic websites using frameworks like Catalyst and Mojolicious.
- Server-side scripting with CGI (common gateway interface).
- Processing form data and generating web pages dynamically.
4. Network Programming
With modules like Net::HTTP and Socket, Perl is well-suited for network-related tasks, including:
- Automating data transfers between servers.
- Writing custom servers or client applications.
- Parsing and analyzing network protocols.
5. Database Interaction
Perl offers robust support for database programming through modules like DBI (database independent interface). It is commonly used for:
- Querying and managing relational databases such as MySQL, PostgreSQL, and Oracle.
- Migrating and transforming database records.
- Automating backup and restore operations.
6. Bioinformatics
Perlโs ability to handle large text files and parse complex data has made it a popular choice in bioinformatics for:
- Analyzing DNA, RNA, and protein sequences.
- Building pipelines for processing biological data.
- Integrating with specialized bioinformatics libraries.
7. Log File Analysis
Perl is commonly employed to extract, process, and analyze log files in various industries, including IT and security. It is particularly useful for:
- Parsing web server logs to generate traffic reports.
- Monitoring security logs for anomalies.
- Summarizing data trends from raw logs.
8. Automation and Scripting
From simple one-liners to comprehensive automation scripts, Perl is a go-to language for:
- Automating repetitive tasks.
- Building cron jobs for scheduled operations.
- Writing test scripts for software development.
9. Prototyping and Proof of Concepts
Perlโs concise syntax and powerful features make it ideal for quickly prototyping applications or creating proof-of-concept solutions.
10. Legacy Application Maintenance
Many legacy systems and scripts are written in Perl, particularly in industries like finance and telecommunications. Perl is used to maintain, update, or migrate these systems.
Advantages and Disadvantages of Using Perl
Perl is a versatile and powerful programming language with a long history of use in various domains, from text processing to web development. Like any tool, it comes with its own set of strengths and weaknesses, which can influence its suitability for specific projects.
What Are the Advantages of Perl?
Perl offers several practical benefits that go beyond its technical features, making it a preferred choice for certain tasks and use cases:
- Quick development time. Perlโs concise syntax and flexibility allow developers to write scripts and programs faster compared to many other languages. This makes it ideal for rapid prototyping and automating small to medium-sized tasks.
- Strong community and ecosystem. Perl benefits from a robust and active community that provides extensive documentation, forums, and a vast library of reusable modules through CPAN. This support ecosystem accelerates development and problem-solving.
- High text-processing efficiency. Perlโs text processing capabilities are unmatched, making it particularly advantageous for tasks like log analysis, web scraping, and data transformation. It can handle large datasets efficiently without requiring additional tools.
- Portability and cross-platform compatibility. Perl runs on a wide range of platforms, including Unix, Linux, macOS, and Windows, without significant modifications to the code. This makes it a great choice for projects requiring portability across systems.
- Versatility in applications. Perl is a general-purpose language capable of addressing diverse use cases, such as system administration, web development, database interaction, and bioinformatics, making it a one-stop solution for many programming needs.
- Backward compatibility. Perl places a strong emphasis on backward compatibility, ensuring that older scripts and programs continue to run smoothly on newer versions of the language. This reduces the maintenance burden for legacy systems.
- Flexible problem-solving approach. Perlโs philosophy of "Thereโs More Than One Way To Do It" allows developers to approach problems in different ways, catering to various coding styles and preferences.
- Free and open source. Perl is open-source software, meaning it is freely available and has no licensing costs. This makes it a cost-effective solution for both personal and commercial projects.
- Proven stability and reliability. With decades of use in production environments, Perl has proven its stability and reliability, making it a trusted language for critical applications.
- Seamless integration with other technologies. Perl can easily interact with other programming languages, tools, and systems, making it ideal for glue code that connects different components or processes.
What Are the Disadvantages of Perl?
While Perl has many strengths, it also comes with limitations and challenges that can affect its suitability for certain projects. Below are some of the key disadvantages of using Perl:
- Readability issues. Perlโs flexibility and permissive syntax, while advantageous for rapid development, can lead to code that is difficult to read and maintain. The โThereโs More Than One Way To Do Itโ philosophy often results in inconsistent coding styles.
- Steeper learning curve. For beginners, Perlโs syntax can be complex and non-intuitive, especially when dealing with regular expressions, context sensitivity, and advanced features like references and typeglobs.
- Performance concerns for large applications. While Perl performs well for scripts and small applications, it may not be as efficient as languages like C++ or Java for large, performance-critical systems due to its interpreted nature.
- Declining popularity. Perlโs popularity has waned in recent years with the rise of newer programming languages such as Python, Ruby, and JavaScript. This decline has led to fewer new developers learning Perl and a smaller pool of talent for hiring.
- Limited modern frameworks. While Perl has frameworks like Catalyst and Mojolicious, they are not as widely adopted or as feature-rich as modern frameworks in other languages, such as Django for Python or Rails for Ruby.
- Verbose legacy code. Older Perl scripts and applications often rely on outdated coding practices, making them cumbersome to update or refactor. Maintaining legacy Perl code can be particularly challenging for developers unfamiliar with its nuances.
- Weak native GUI support. Perl lacks robust native support for building graphical user interfaces (GUIs), making it less suitable for developing desktop applications compared to languages like Java or C#.
- Dependency management complexity. Managing dependencies via CPAN can sometimes introduce challenges, particularly when dealing with module versioning or platform-specific compatibility issues.
- Concurrency and multithreading limitations. While Perl does support threading, its concurrency model is less mature and performant compared to languages like Go or Java, making it less ideal for multithreaded applications.
- Outdated perception. Perl is often perceived as a "dated" language, which can deter businesses and developers from choosing it for new projects, even when it may be suitable for the task.
Perl FAQ
Here are the answers to the most commonly asked questions about Perl.
Is Perl Frontend or Backend?
Perl is primarily a backend programming language, used for server-side tasks such as data processing, system administration, web development, and database interaction. It excels in handling backend operations like parsing files, automating scripts, and managing server processes. While Perl can be used in web development to generate dynamic content through frameworks like Catalyst or Mojolicious, it is not typically used for frontend development tasks like designing user interfaces or implementing client-side interactivity, which are better suited to languages like JavaScript, HTML, and CSS.
Why Do Hackers Use Perl?
Hackers often use Perl due to its flexibility, powerful text manipulation capabilities, and portability. Perl's robust support for regular expressions allows hackers to efficiently search, modify, and analyze text, such as parsing logs or extracting sensitive information from data files. Its ability to handle complex tasks with concise scripts makes it ideal for automation, penetration testing, and creating exploits. Additionally, Perl's compatibility with various operating systems enables hackers to write code that works seamlessly across platforms. The availability of extensive libraries and modules on CPAN further simplifies tasks like network scanning, cryptography, and interacting with system resources, making Perl a valuable tool for both ethical and malicious hacking activities.
What Is the Future of Perl?
The future of Perl is a mix of stability and niche relevance, with a focus on maintaining its role in legacy systems, specialized domains, and scripting tasks. While Perl's popularity has declined due to competition from more modern languages like Python, Ruby, and Go, it remains a reliable choice for tasks requiring strong text processing and system automation. Its extensive library ecosystem (CPAN), backward compatibility, and active core development ensure that Perl will continue to serve industries reliant on its existing codebases.
Efforts to modernize Perl, such as ongoing updates to the language and frameworks, aim to keep it relevant for enthusiasts and specialized applications. However, its future growth will likely be limited to specific domains like bioinformatics, network programming, and legacy system maintenance rather than becoming a mainstream choice for new projects.