Algorithms are step-by-step procedures or formulas for solving problems or performing tasks. They are fundamental to computer science and mathematics, enabling efficient data processing, calculations, automated reasoning, and other computational tasks.
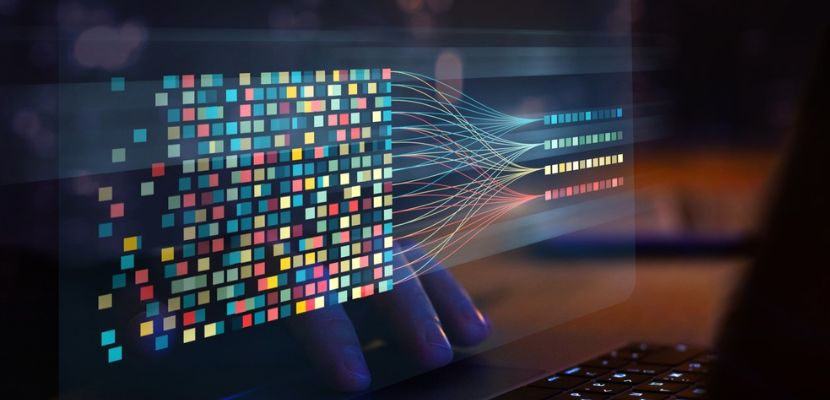
What Is an Algorithm?
An algorithm is a precise sequence of well-defined instructions designed to perform a specific task or solve a particular problem. It operates within a finite amount of time and uses a finite amount of resources, such as memory and computational power. Algorithms are fundamental to computer science and mathematics, providing the underlying logic that drives software and hardware systems. They can range from simple processes, like adding two numbers, to complex operations, such as those found in artificial intelligence and cryptography.
An algorithm begins with an initial state and follows a series of steps to achieve a desired end state or output. Each step in an algorithm is typically straightforward and unambiguous, ensuring that it can be implemented consistently. The efficiency of an algorithm is a critical aspect, often evaluated based on time complexity (how the execution time scales with the size of the input) and space complexity (how the memory requirement scales with the size of the input).
Algorithms are used in a vast array of applications, from everyday tasks like searching and sorting data to more advanced uses in fields such as data analysis, machine learning, and network security. The study and design of algorithms are central to advancements in technology and are integral to problem-solving in numerous scientific and engineering disciplines.
How Do Algorithms Work?
Algorithms work by following a series of well-defined steps to perform tasks or solve problems. Here's a detailed explanation of how they operate:
- Input. Algorithms start with an input, which can be any data or information that the algorithm needs to process. Inputs range from simple numerical values to complex data structures like lists, graphs, or databases.
- Step-by-step instructions. The core of an algorithm consists of a sequence of specific, unambiguous instructions. These instructions guide the algorithm through a series of actions, which can include mathematical calculations, data manipulation, decision-making processes, and more.
- Processing. As the algorithm executes, it processes the input data according to the defined instructions, such as arithmetic or logical operations.
- Intermediate states. During its execution, an algorithm may go through multiple intermediate states, where it temporarily stores and updates data. These states are essential for tracking progress and ensuring that the algorithm can move from one step to the next.
- Output. After processing the input data, the algorithm produces an output. The output is the result of the algorithm's computations and is typically the solution to the problem or the completion of the task for which the algorithm was designed. Outputs can vary widely, from numerical results to sorted lists, and from Boolean values (true/false) to complex data structures.
- Termination. A well-designed algorithm has a clear termination point, meaning it knows when to stop. This ensures that the algorithm doesn't run indefinitely and that it completes its task within a reasonable time frame. Termination is achieved when the algorithm reaches its final step or when a specific condition is met.
- Correctness and efficiency. An algorithm is correct if it produces the expected output for all valid inputs. This means it must handle all possible cases and edge scenarios accurately. The efficiency of an algorithm is measured by how well it uses resources, such as time and memory. An efficient algorithm performs its task quickly and with minimal resource consumption. Efficiency is often analyzed using concepts like time complexity and space complexity.
Algorithm Characteristics
Algorithms possess several key characteristics that define their functionality and efficiency. Here are the primary attributes algorithms must have to perform their tasks correctly, efficiently, and reliably:
- Correctness. An algorithm must produce the correct output for all valid inputs. This means it should handle all possible cases, including edge cases, and yield the expected results consistently. Correctness is essential for the reliability of an algorithm.
- Efficiency. Efficiency refers to how well an algorithm uses resources, such as time and memory. It is typically analyzed through time complexity (how execution time scales with input size) and space complexity (how memory usage scales with input size). Efficient algorithms perform tasks faster and with less resource consumption.
- Finiteness. An algorithm must have a finite number of steps. It should reach a conclusion after a limited number of operations, ensuring that it doesn't run indefinitely. This characteristic guarantees that the algorithm will terminate and produce a result.
- Definiteness. Each step of an algorithm must be precisely defined and unambiguous. The instructions should be clear and understandable, leaving no room for interpretation. This ensures that the algorithm can be implemented correctly and consistently.
- Input. Algorithms typically start with an input, which is the data or information they need to process. The input can be simple or complex, but it must be well-defined and provided at the beginning of the algorithm.
- Output. An algorithm should produce an output, which is the result of its computations. The output must be clearly defined and related to the input, providing the solution to the problem or completing the specified task.
- Generality. An algorithm should be general enough to solve a broad class of problems, not just a specific instance. This characteristic ensures that the algorithm is versatile and can be applied to various inputs and scenarios within its problem domain.
- Scalability. A scalable algorithm can handle increasing amounts of data or larger problem sizes efficiently. Scalability is crucial for algorithms used in environments where data volume or complexity grows over time.
- Robustness. A robust algorithm can handle unexpected situations, such as invalid inputs or errors, gracefully. It should have mechanisms to deal with anomalies and continue functioning correctly or terminate gracefully with an appropriate error message.
Types of Algorithms
Algorithms come in various types, each designed to solve different kinds of problems and perform specific tasks. Understanding the types of algorithms helps in choosing the right approach for a given problem. Here are some common types of algorithms.
Sorting Algorithms
- Bubble sort. This is a simple comparison-based algorithm where each pair of adjacent elements is compared, and the elements are swapped if they are in the wrong order. The process is repeated until the list is sorted.
- Quick sort. Uses a divide-and-conquer strategy to partition the array into smaller sub-arrays and then sorts them. It is efficient and commonly used.
- Merge sort. Another divide-and-conquer algorithm that splits the array into halves, sorts them, and then merges the sorted halves. It ensures stable sorting and has a predictable time complexity.
Search Algorithms
- Linear search. Scans each element in a list sequentially until the desired element is found or the list ends. It is simple but inefficient for large lists.
- Binary search. Efficiently searches a sorted list by repeatedly dividing the search interval in half. It has a logarithmic time complexity, making it much faster than linear search for large datasets.
Dynamic Programming Algorithms
- Fibonacci sequence. Computes the Fibonacci numbers by storing the results of subproblems to avoid redundant calculations. This approach significantly reduces the time complexity.
- Knapsack problem. Solves optimization problems by breaking them down into simpler subproblems and storing the results to avoid redundant work, making it suitable for resource allocation problems.
Greedy Algorithms
- Dijkstra's algorithm. Finds the shortest path from a starting node to all other nodes in a weighted graph by always choosing the shortest edge.
- Huffman Coding. Used for data compression, it builds an optimal prefix tree that minimizes the total length of encoded data by using a greedy approach.
Backtracking Algorithms
- N-Queens problem. Places N queens on an N×N chessboard so that no two queens threaten each other. It tries out different configurations and backtracks upon encountering conflicts.
- Sudoku solver. Solves the Sudoku puzzle by trying out numbers in empty cells and backtracking when a contradiction is found.
Divide and Conquer Algorithms
- Merge sort. It divides the array into halves, recursively sorts them, and then merges the sorted halves.
- Quick sort. Also uses divide-and-conquer by selecting a pivot element, partitioning the array around the pivot, and then recursively sorting the partitions.
Recursive Algorithms
- Factorial calculation. Computes the factorial of a number using recursive calls to break down the problem into smaller subproblems.
- Towers of Hanoi. Solves the puzzle by recursively moving disks between rods, demonstrating a classic example of recursion.
Graph Algorithms
- Breadth-first search (BFS). Explores all nodes at the present depth level before moving on to nodes at the next depth level, useful for finding the shortest path in unweighted graphs.
- Depth-first search (DFS). Explores as far down a branch as possible before backtracking, useful for exploring all possible paths in a graph.
String Algorithms
- Knuth-Morris-Pratt (KMP) algorithm. Searches for a substring within a string by preprocessing the pattern to avoid redundant comparisons.
- Rabin-Karp algorithm. Uses hashing to find any one of a set of pattern strings in a text, efficiently detecting matches.
Machine Learning Algorithms
- Linear regression. Models the relationship between a dependent variable and one or more independent variables using a linear equation.
- K-means clustering. Partitions a dataset into K clusters by minimizing the variance within each cluster, used for unsupervised learning tasks.
Uses of Algorithms
Algorithms are fundamental to many fields, providing solutions to various problems and performing a wide range of tasks. Here are some key uses of algorithms:
- Data sorting. Algorithms like quick sort, merge sort, and bubble sort are used to organize data in a specific order, which is essential for efficient data retrieval and processing.
- Search operations. Linear search and binary search algorithms help find specific elements within data structures. They are crucial in databases and search engines for quickly locating information.
- Optimization problems. Algorithms such as dynamic programming (e.g., the knapsack problem) and greedy algorithms (e.g., Dijkstra’s algorithm) are used to find the best solution among many possible options, optimizing resource allocation and decision-making processes.
- Cryptography. Encryption and decryption algorithms ensure data security and privacy. Algorithms like RSA and AES are used to protect sensitive information in communication and storage.
- Pathfinding and navigation. Graph algorithms like breadth-first search (BFS) and A* are used in navigation systems and robotics to find the shortest or most efficient path from one point to another.
- Machine learning and data mining. Algorithms such as linear regression, decision trees, and K-means clustering are used in the fields of artificial intelligence and data science to analyze data, make predictions, and identify patterns.
- Compression. Algorithms like Huffman coding and LZW (Lempel-Ziv-Welch) are used to reduce the size of data for efficient storage and data transmission, essential in multimedia and communication technologies.
- Image and signal processing. Algorithms are used to enhance, compress, and analyze images and signals. For example, Fast Fourier Transform (FFT) algorithms are used in audio and signal processing to convert signals from time domain to frequency domain.
- Network and web services. Algorithms manage and optimize the flow of data across networks, ensuring efficient and reliable communication. They also power search engines, recommendation systems, and social media platforms.
- Biological computation. Algorithms are used in bioinformatics to analyze biological data, such as DNA sequencing and protein structure prediction, aiding in medical research and biotechnology.
- Financial modeling and trading. Algorithms are employed in financial markets to predict trends, assess risks, and execute high-frequency trading, enabling more informed investment decisions and efficient market operations.
- Robotics and automation. Control algorithms guide the movement and operations of robots, ensuring precise and efficient performance in tasks ranging from manufacturing to medical surgery.
- Game development. Pathfinding and AI algorithms enhance the intelligence and realism of non-player characters (NPCs) in video games, creating more engaging and challenging gameplay experiences.
- Natural language processing (NLP). Algorithms in NLP help computers understand, interpret, and generate human language, enabling applications like language translation, sentiment analysis, and voice-activated assistants.
- Weather forecasting and climate modeling. Complex algorithms analyze meteorological data to predict weather patterns and model climate changes, aiding in disaster preparedness and environmental conservation.
How Are Algorithms Analyzed?
Algorithm analysis primarily focuses on evaluating the efficiency and correctness of algorithms.
Efficiency is typically measured in terms of time complexity and space complexity. Time complexity assesses how the execution time of an algorithm scales with the size of the input, often expressed using Big O notation (e.g., O(n), O(log n), O(n^2)), which describes the upper bound of the algorithm's growth rate. Space complexity evaluates how much memory the algorithm requires relative to the input size.
Correctness ensures that the algorithm produces the right output for all valid inputs, often verified through formal proofs or extensive testing.
Other considerations include stability (whether the algorithm preserves the order of equal elements), robustness (its ability to handle edge cases and unexpected inputs), and scalability (how well it performs as the input size grows). By analyzing these aspects, developers can choose the most suitable algorithms for specific tasks, ensuring optimal performance and reliability.
How to Design an Algorithm?
Designing algorithms involves a systematic approach to problem-solving that includes several key steps. Here’s a detailed overview:
- Problem definition. Clearly understand and define the problem that needs to be solved. This involves identifying the inputs, desired outputs, and any constraints or requirements.
- Planning and strategy selection. Determine the most suitable strategy or paradigm to tackle the problem. Common strategies include divide-and-conquer, dynamic programming, greedy algorithms, and backtracking. Selecting the right approach depends on the nature of the problem and the efficiency requirements.
- Algorithm design. Break down the problem into smaller, manageable parts. Outline the step-by-step procedure for solving each part. Use pseudocode or flowcharts to map out the algorithm’s logic and structure. This stage focuses on creating a high-level representation of the algorithm without delving into specific programming languages.
- Detailed specification. Convert the high-level design into detailed instructions. Define the exact sequence of operations, including loops, conditionals, and data manipulations. Ensure that each step is precise and unambiguous.
- Implementation. Translate the detailed algorithm into a specific programming language. Write the code following best practices for readability, maintainability, and efficiency. During implementation, consider edge cases and error handling to ensure robustness.
- Testing and verification. Test the algorithm with various inputs, including edge cases and typical scenarios, to verify its correctness and efficiency. Use both unit tests (testing individual components) and integration tests (testing the algorithm as a whole) to ensure comprehensive coverage.
- Optimization. Analyze the performance of the algorithm and identify any bottlenecks or inefficiencies. Optimize the code to improve time complexity and space complexity. This may involve refining the logic, improving data structures, or implementing more efficient algorithms for specific tasks.
- Documentation. Document the algorithm thoroughly, including explanations of the logic, design choices, and usage instructions. Good documentation aids in future maintenance, debugging, and understanding by other developers.
- Review and iteration. Review the algorithm with peers or mentors to get feedback and identify potential improvements. Based on feedback and new insights, iterate on the design, implementation, and testing phases.