Converting a string to an integer is a common operation in Java. It helps process user input, such as data from text files or web applications.
Java has multiple ways to do this conversion. Each method has its use case and benefits.
This guide shows several ways to convert strings to integers in Java.
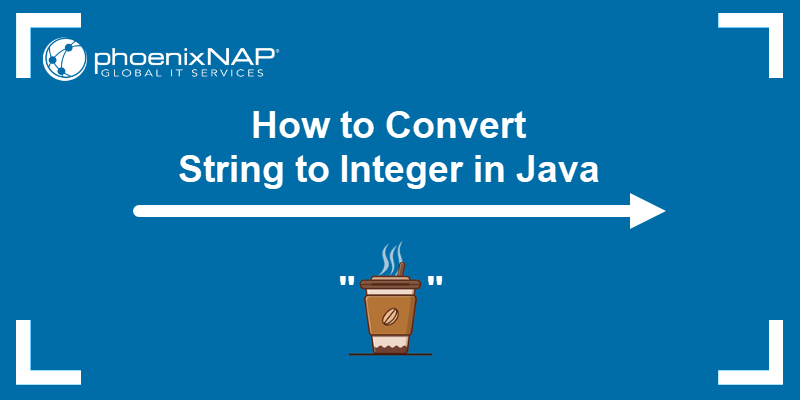
Prerequisites
- Java installed (JDK) to run and compile examples (This guide uses Java 21).
- Access to the command line or Java IDE to write and test the code.
Note: To install Java, follow one of our guides:
Converting Strings to Integers in Java
Java has several ways to process strings and convert them into integers. Below, we outline three different methods and show code examples.
Convert String to Integer Using Integer.parseInt()
The simplest way to convert a string to an integer is using the Integer.parseInt()
method. It converts a string to a primitive int
. For example:
String numberString = "123";
int numberInt = Integer.parseInt(numberString);
If the string is not an integer, the method throws NumberFormatException
. Use a try-catch block to handle the exception:
try {
String numberString = "abc";
int numberInt = Integer.parseInt(numberString);
System.out.println("Result: " + numberInt);
} catch (NumberFormatException e) {
System.out.println("Caught exception: " + e.getMessage());
}
The block helps handle invalid inputs.
Convert String to Integer Using Integer.valueOf()
To convert a string to an Integer
object, use the Integer.valueOf()
method instead. For example:
String numberString = "123";
Integer numberInteger = Integer.valueOf(numberString);
For an invalid string, the method also throws a NumberFormatException
. A try-catch block helps handle the exception:
public class StringToIntDemo {
public static void main(String[] args) {
try {
String numberString = "abc";
Integer numberInteger = Integer.valueOf(numberString);
System.out.println("Result: " + numberInteger);
} catch (NumberFormatException e) {
System.out.println("Caught exception: " + e.getMessage());
}
}
}
Use this method if working with collections or nullable values.
Convert String to Integer Using Ints Method
If using the Guava library, try the Ints.tryParse()
method. For example:
import com.google.common.primitives.Ints;
String numberString = "123";
Integer numberInt = Ints.tryParse(numberString);
The method returns null on an invalid input. Use it in cases where exceptions are costly.
Note: To use Guava, download the Guava JAR or add the dependency to your build tool (Maven, Gradle, etc.).
Convert to Integer or Int?
The choice of whether to convert to an Integer or int depends on the use case:
int
. Use when you're sure there is a value present. It is a primitive type that's used for faster performance and memory efficiency.Integer
. Use the wrapper class for collections, null values, or object semantics.
Conclusion
This guide showed how to convert Java strings to integers. Use a method that best fits your coding style and use case.
Next, learn more about object-oriented programming design using SOLID principles.