Introduction
PHP is a server-side scripting language used in web development. PHP scripts automate tasks and introduce new functions and features to websites and applications.
If a script encounters an issue that prevents it from executing a task, PHP can display errors on the webpage or log error messages to a file.
Learn how to enable and configure PHP Error Reporting and use error logs to optimize your web development projects.
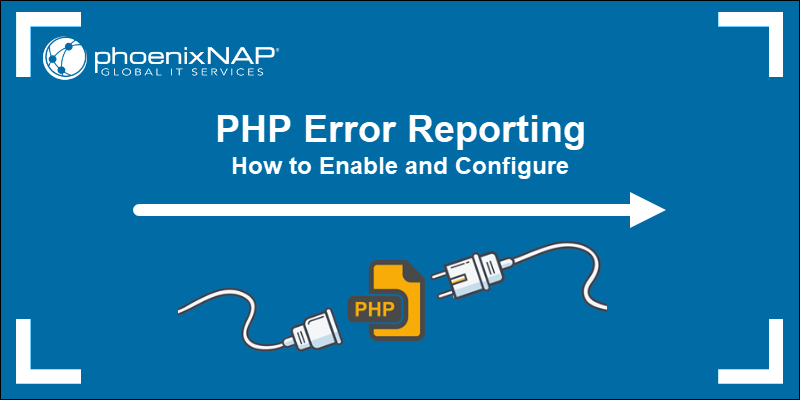
Prerequisites
- PHP installed.
- Permissions to access and edit web server configuration files.
- Access to a command line/terminal window.
How to Enable PHP Error Reporting in PHP Script
A PHP error occurs when there is an issue within the PHP code. For example, using incorrect syntax or forgetting a semicolon prompts a notice, while calling an improper variable can lead to a fatal error that halts the execution of a script.
Users can configure their PHP scripts and server configuration files to display these errors directly on a website or log them in a file.
Warning: Displaying errors on a webpage helps debug issues during the development process. However, you should avoid displaying errors on the website in production environments and only log errors to a secure file. The error message may reveal server configuration settings, sensitive code, or other information that poses a cybersecurity risk.
Enable PHP Error Reporting in php.ini File
Settings in the php.ini configuration file control PHP's behavior regarding error display and logging. In many production environments, these settings are turned off by default.
To enable error reporting in the php.ini file:
1. Locate the php.ini file. The path to the file varies based on the PHP version, server type, and Linux distribution. Look for the file in the PHP installation directory, within the PHP version and server type subdirectory. For example, the default php.ini path for PHP 8.1 running on an Apache server is:
/etc/php/8.1/apache2/php.ini
2. Open the php.ini file using a preferred text editor. The following command uses nano to access the file:
sudo nano /etc/php/8.1/apache2/php.ini
Replace the path with the file path on your system.
3. To display errors on a webpage, set the display_errors
directive to On
. This setting should only be used in development or testing environments. To avoid sensitive information leakage, set the value to Off
in production environments.
4. Confirm that the log_errors
directive is set to On
.
5. Specify the path to the error log file using the error_log
directive. Uncomment the line by removing the semicolon (;
):
error_log = /path/to/your/webserver/php_error.log
Ensure the specified directory exists and the user has permissions to write to the file.
6. Save the changes and exit the file.
7. Restart the Apache server to ensure the changes have been applied.
sudo systemctl restart apache2
Depending on the setup, PHP errors are now visible in the error log or webpage. Try introducing a deliberate mistake in your PHP code and execute the script in a testing environment to see if the error appears.
Configure Error Reporting in PHP Script
It is not always practical to edit global PHP settings in the php.ini file, especially in shared hosting environments where settings might affect other applications.
PHP has additional error and logging functions that can be inserted directly into PHP scripts. Use these runtime functions to control how errors are handled and logged in each script without editing the php.ini file.
Display PHP Errors Using ini_set
The ini_set
runtime function allows you to change error reporting settings dynamically during script execution. This means that the settings in the php.ini file do not need to be configured in advance for these functions to execute.
To configure a PHP script to display all errors on the webpage, insert the following lines at the beginning of your PHP code file:
<?php
ini_set('display_errors', '1');
ini_set('error_reporting', E_ALL);
?>
To ensure all PHP errors are displayed, including those that occur during PHP's startup sequence, enter:
<?php
ini_set('display_errors', '1');
ini_set('display_startup_errors', '1');
?>
Use the following code to disable error display on live websites and protect sensitive information:
<?php
ini_set('display_errors', '0');
?>
To enable error logging to a server file without displaying errors to end users, use:
<?php
ini_set('log_errors', '1');
?>
Errors are logged to the default server log file or a specific file if the error_log
path is set in the php.ini file.
Control Error Level with error_reporting
The error_reporting()
function allows developers to define which PHP errors are reported. Whether these errors are displayed on the webpage or logged in an error file is controlled by the display_errors
and log_errors
directives in the php.ini file.
To define the level of error reporting within a specific PHP script, add the error_reporting()
runtime function at the beginning of the PHP file:
<?php
// Report all errors
error_reporting(E_ALL);
?>
The E_ALL
directive instructs PHP to report all errors, including warnings, fatal errors, and notices.
Use the OR (|
) bitwise operator to report specific types of errors. For example, add the following code snippet at the beginning of the PHP script to display fatal errors, warnings, and parse errors:
<?php
// Report only fatal, warning, and parse errors
error_reporting(E_ERROR | E_WARNING | E_PARSE);
?>
Use the not symbol (~
) to omit specific error types from reporting. For example, to report all errors except notices, enter:
<?php
// Report all errors except notices
error_reporting(E_ALL & ~E_NOTICE)
?>
To turn off error reporting in PHP, set the error_reporting
value to 0
:
<?php
error_reporting(0);
?>
Ensure that the display_errors
directive value in the php.ini file is set to 0
, or use the ini_set
function to dynamically disable this directive before using error_reporting
in production environments.
Customize Error Reporting Messages via error_log
The error_log
function can fine-tune error-handling mechanisms and send custom messages to local server error logs or a specific email address.
Log a custom error message to the default error log by adding the following code to your PHP script:
$message = "Custom error message";
error_log($message);
To redirect error messages to a custom error log file, set the message type to 3
and enter the path to the custom file:
$message = "Custom error message";
error_log($message, 3, "/path/to/custom_error.log");
Set the message type to 1
, and enter the recipient's email address to send error messages via email:
$message = "Custom error message";
error_log($message, 1, "[email protected]");
This method is practical when alerting specific groups or individuals to critical errors by reducing response times.
How to Log Errors via Web Server Configuration
Logging PHP errors through web server configuration allows you to monitor PHP errors within an application without altering its PHP code.
Note: Back up configuration files before making changes. Editing server configuration files affects your entire server and all hosted websites.
Enable PHP Error Logging via .htaccess
An .htaccess file can be configured to enable PHP error logging for a specific directory. This method does not require changing the default server configuration and applies to Apache web servers.
To enable PHP error logging via an .htaccess file:
1. Locate an existing .htaccess file or create a new one in the same directory as the PHP file. Settings in the .htaccess file affect this directory and all subdirectories.
Note: Learn how to enable and set up a .htaccess file in Apache.
2. Open the file using a text editor and add the following logging directives:
php_flag log_errors On
php_value error_log /path/to/your/php_errors.log
Replace the path in the example with the actual path to the error log file on your system.
3. Save the changes and close the .htaccess file.
4. Use the chmod command to change the permissions and give the web server process read and write access:
sudo chmod 644 /path/to/your/php_errors.log
This command grants read and write access to the file owner and read-only access to others. The directory containing the log file must also have appropriate permissions to allow file creation and modification by the web server.
Log PHP Errors via Apache Server Configuration
Developers who prefer a server-wide configuration or do not want to edit the .htaccess file can modify the main Apache configuration file instead. Depending on the Linux distribution, Apache settings are usually configured in the httpd.conf or apache2.conf file.
To enable PHP error reporting in the Apache configuration file:
1. Open the httpd.conf or apache2.conf file using a text editor:
For Debian/Ubuntu systems, use:
sudo nano /etc/apache2/apache2.conf
For other Linux distributions, enter:
sudo nano /etc/httpd/httpd.conf
2. Add or modify the ErrorLog
directive to specify the path to the error log:
ErrorLog "/path/to/your/log/website-error.log"
Replace the path in the example with the log file path on your system.
3. Restart the Apache server for the changes to take effect:
For Debian/Ubuntu systems:
sudo systemctl restart apache2
Other systems:
sudo systemctl restart httpd
PHP error logging via the Apache server configurations also requires correct settings in the php.ini file. Check if the log_errors
directive is enabled and the error_log
directive points to the correct log file.
How to Send PHP Error Log via Email
Emails are an efficient way to send immediate alerts when an issue occurs on a server or website. Use this option cautiously to avoid sending a large number of emails and increasing the load on server resources.
Note: Apply encryption and secure email practices when sharing sensitive information over an email service.
Using Custom PHP Error Handler
The following PHP function logs error messages and sends them via the error_log()
function to a specified email address.
Insert the following code at the beginning of the PHP script where you want to apply custom error handling:
function customError($errno, $errstr, $errfile, $errline) {
$errorMsg = "Error: [$errno] $errstr\nFile: $errfile\nLine: $errline";
// Set email headers
$headers = "From: [email protected]\r\n";
// Use error_log() to send emails
error_log($errorMsg, 1, "[email protected]", $headers);
// Check if displaying errors is enabled and output the error message if it is
if (ini_get('display_errors')) {
echo $errorMsg;
}
}
// Register custom error handler
set_error_handler("customError");
The code formats the error message to include the error number, string, the file in which the error occurred, and the line number. Include this script early in the execution flow to ensure it catches as many runtime errors as possible.
Using the error_log Function
The error_log
function logs and sends PHP error messages to email addresses or remote servers. It is straightforward to implement and, as explained earlier, can be used to send an error message to an email address for specific errors.
However, the php.ini configuration does not natively support emailing error logs, and it does not provide comprehensive error logging or detailed analytics.
In production environments, developers need to supplement the error_log
function with:
- Monitoring Tools and Scripts. After logging errors to a file via the
error_log
function, developers can apply an external tool or script that periodically checks the error log file and sends emails if new errors are found. These tools and scripts can also register errors logged by PHP itself, broadening the scope of logged errors. - Dedicated Logging Services. Dedicated logging services are comprehensive third-party solutions that can handle and filter extensive error logs from multiple sources. External solutions allow developers to aggregate logs, receive real-time analysis, and get notified about important issues through alerts or emails.
The php.ini configuration file is essential for enabling PHP Error reporting, especially in development scenarios. Applications in a production environment need additional tools and services to automate and enhance error logging.
Conclusion
This tutorial has provided several alternatives to enabling and showing PHP errors and warnings. By receiving quick and accurate error notifications, you can now troubleshoot PHP issues more efficiently.
The 403 Forbidden error is common after setting up a website using Apache. Learn why your webpage won't load and how to fix this error.