Introduction
Dictionaries are one of Python's most versatile and essential data types. Dictionaries play a crucial role in dynamic data storage through key-value pairs. This data type maps keys to corresponding values and provides fast data access.
In this article, you will learn the basic concepts and advanced techniques of Python dictionaries through practical examples.
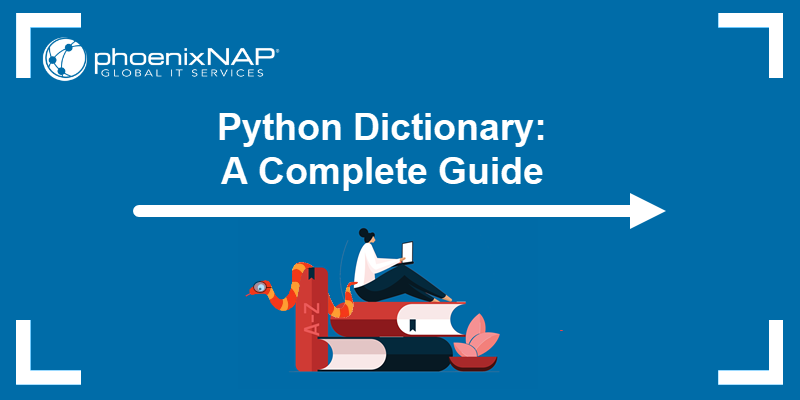
Prerequisites
- A working Python 3 installation.
- An IDE or text editor for Python code examples.
What Is a Dictionary in Python?
A dictionary in Python is a built-in data type of unordered key-value pairs. The data type connects related pieces of data into pairs, associating every key with a corresponding value. Every key is unique and helps retrieve an associated value.
Python dictionaries are:
- Unordered. Items in a Python dictionary are not persistent in Python versions below 3.7. The order in which items were added to the dictionary is not guaranteed when retrieving data from a dictionary. Starting in Python 3.7, the insertion order remains the same.
- Mutable. Mutability enables adding, removing, and modifying items in the dictionary after creation. Updating items in a dictionary enables changing data as needed.
- Dynamic. A dictionary's size is dynamic. The data type expands as more items are added and shrinks when removing items. Dynamic resizing ensures memory efficiency when handling varying amounts of data.
- Heterogenous. Keys and values in a dictionary can be different data types, including dictionaries. Dictionary keys are unique, immutable, and use a hashable value.
Python Dictionary Example
A dictionary contains items with key-value pairs. This data type is convenient for storing pairs of related information into a single data item.
An example dictionary looks like the following:
worker = {
"name": "John",
"age": 30,
"position": "Software Engineer",
"languages": ["Python", "Java", "C++"],
"projects": {
"project 1": "Web applications",
"project 2": "Android applications",
"project 3": "Machine learning"
}
}
print(worker)
The example shows how complex information, such as worker data, is simple to represent using a Python dictionary.
How to Create a Dictionary in Python?
The simplest way to create a Python dictionary is to add a comma-separated sequence of elements between curly braces. Separate key-value pairs with a colon, like in the example below:
my_dictionary = {"one": 1, "two": 2, "three": 3}
There are several different ways to initialize a Python dictionary, which provides flexibility for different use cases and programming styles.
Create a Dictionary with Integer Keys
A dictionary with integer keys helps represent sequential data. The example below demonstrates how to create integer keys in Python:
integer_keys = {
1: "one",
2: "two",
3: "three"
}
print(integer_keys)
Integer keys are simple to iterate over and are smaller in memory than other data types.
Create a Dictionary with Mixed Keys
Dictionary keys do not have to be the same data type. A key can be any immutable data type, such as a string, integer, float, or tuple. The example below demonstrates how to create a dictionary with mixed keys:
mixed_keys = {
"one": 1,
2: 2,
3.0: 3,
(4,1): 4
}
print(mixed_keys)
Mixed keys provide flexibility and are helpful when dealing with multiple data type inputs. However, the approach should be used infrequently as the code gets harder to read and introduces additional complexity.
Note: Learn about Python dictionary comprehension, a technique for creating Python dictionaries in one line.
Working With Dictionaries in Python
Several operations enable viewing and controlling a dictionary's contents. Basic dictionary tasks include:
- Accessing dictionary items.
- Adding new items.
- Updating existing elements.
- Removing items.
The sections below demonstrate how to perform each of these operations.
Accessing Python Dictionary Values
To access a Python dictionary value, provide the appropriate key as an index. The syntax is:
dictionary[key]
For example, the code below shows how to create and access a specific element from the dictionary:
my_dictionary = {"one": 1, "two": 2, "three": 3}
print(my_dictionary["one"])
print(my_dictionary["two"])
print(my_dictionary["three"])
The code returns an error if a key does not exist in the dictionary. To avoid the error, use the get()
method, which allows returning a value in cases where the key does not exist. For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
print(my_dictionary.get("four", "Not found"))
Since the key "four"
is undefined in the dictionary, the method returns the "Not found"
message. The get()
method is convenient when a dictionary runs through a loop or program, which should return a value instead of an error.
Adding to Python Dictionary
There are several different ways to add items to a Python dictionary. The simplest is to use the assignment operator to provide a new key and value. The syntax is:
dictionary[key] = value
For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
my_dictionary["four"] = 4
print(my_dictionary)
Note: The process overwrites the existing value if the key exists in the dictionary.
Other methods allow advanced operations while adding items, including adding multiple items or checking if a key exists to avoid overwriting.
Updating Python Dictionary Elements
Update a Python dictionary value by reassigning a new value to an existing key. The syntax is identical to adding items, except the key exists in the dictionary. For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
my_dictionary["one"] = 0
print(my_dictionary)
The dictionary updates to the new value for the provided key.
Removing Items From Python Dictionary
To remove key-value pairs from a Python dictionary, use the del
statement and specify the associated key. The syntax is:
del dictionary[key]
For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
del my_dictionary["one"]
print(my_dictionary)
If the key does not exist in the dictionary, the statement results in an error.
Other Python Dictionary Methods
Python dictionaries work with many practical methods to perform various operations. The additional operations make dictionaries a versatile data type suitable for many programming problems.
A few of the most critical dictionary methods are described in the sections below.
copy() Method
The copy()
method produces a shallow copy of a dictionary. After creating a copy, adding new items to the original dictionary does not reflect on the copy. However, modifying elements in a copy with a nested dictionary modifies the original.
The code below demonstrates how to use the copy()
method on a dictionary and the behavior of shallow copies when working with nested dictionaries:
my_dictionary = {"one": 1, "two": 2, "three": {1:1, 2:2}}
my_copy = my_dictionary.copy()
print("Copied dictionary:\n", my_copy)
my_copy["three"][1] = 0
print("Original after modifying the copy:\n", my_dictionary)
Changing values of nested elements or adding new elements to a nested dictionary copy modifies the original.
len() Method
The len()
method is a built-in Python method that determines the length of a dictionary. The method counts how many key-value pairs are in a dictionary and returns an integer value.
For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
print(len(my_dictionary))
The example code has three key-value pairs, and the method returns the number. The built-in len()
method determines list lengths in Python and other data types.
items() Method
The items()
method provides a view of key-value pairs as a list of tuple pairs. Modifications to the dictionary reflect on the view automatically.
The example below demonstrates this behavior:
my_dictionary = {"one": 1, "two": 2, "three": 3}
my_view = my_dictionary.items()
print(my_view)
my_dictionary["four"] = 4
print(my_view)
Adding a new item to the original dictionary is visible in the items()
view, as are any modifications to existing elements.
setdefault() Method
The setdefault()
method returns a value for the provided key. If the key does not exist in the dictionary, the method returns None
and adds it as a value for the provided key.
The method also takes an optional argument for the value. If the key is not in the dictionary, it immediately adds the provided key-value pair.
For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
# Returns value for existing key
print("Key exists:\n", my_dictionary.setdefault("one"))
# Returns 5 and adds "five":5 to the dictionary
print("Key does not exist:\n", my_dictionary.setdefault("five", 5))
print(my_dictionary)
If a key exists, the setdefault()
method does not modify the dictionary even with the provided value.
Note: Lines starting with #
are Python comments and do not execute in the code.
fromkeys() Method
The fromkeys()
method makes a new dictionary from a provided key-value sequence. The method reads the element keys from a given dictionary and adds provided values (or None
if omitted).
For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
# Values None
print(my_dictionary.fromkeys(my_dictionary))
# New Values
print(my_dictionary.fromkeys(my_dictionary, 1))
When adding a mutable type as a value (such as a list or another dictionary), changing the original mutable data also updates the dictionary value. The example below demonstrates this behavior:
my_dictionary = {"one": 1, "two": 2, "three": 3}
value_list = [0]
new_dictionary = dict.fromkeys(my_dictionary, value_list)
value_list.append(1)
print(new_dictionary)
The resulting dictionary tracks updates made to the values list.
keys() Method
The keys()
method creates a view of a dictionary's keys. For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
print(my_dictionary.keys())
Modifying the original dictionary also changes the view generated by the keys()
method.
values() Method
The values()
method provides a view of a dictionary's values. For example:
my_dictionary = {"one": 1, "two": 2, "three": 3}
print(my_dictionary.values())
The values()
method is similar to the keys()
and items()
behavior. Changing the original dictionary also modifies the generated view.
Python Dictionary Commands and Methods PDF
We've provided a handy PDF cheat sheet to easily remember all the Python dictionary commands and methods covered in this guide. Click the button below to download and use the PDF.
Conclusion
After reading this guide, you learned about Python dictionaries and how to use this versatile data type. Dictionaries are unique key-value pair data types in Python convenient for various programming tasks requiring linked data.
Next, learn how to import and prettyprint JSON files in Python.