Introduction
Dictionaries are a key-value pair data type in Python. The robust data type is essential and optimal for various programming use cases.
Mastering different techniques for initializing a dictionary is crucial for streamlining code for various applications.
This article covers seven different methods to initialize Python dictionaries.
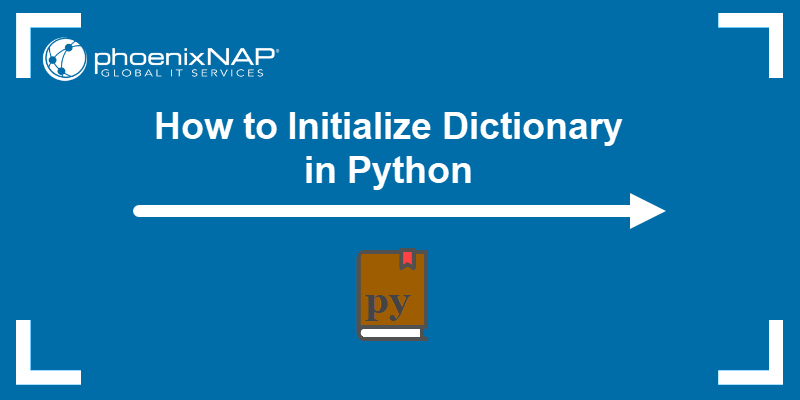
Prerequisites
- Python 3 interpreter installed (see how to check the Python version).
- A functional Python environment (an IDE or text editor).
- Access to the command line/terminal.
Initialize Dictionary in Python: 7 Methods
There are several different methods to initialize a dictionary in Python. Every method is suitable for specific scenarios and programming styles. The sections below provide example codes for every method.
Method 1: Passing Key-Value Pairs as Literals
The simplest and most common way to initialize a Python dictionary is to pass key-value pairs as literals in curly braces. For example:
my_dictionary = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
Use this method when working with a small number of key-value pairs that require straightforward initialization.
Method 2: Using dict() constructor
The built-in dict()
constructor creates a Python dictionary. Pass the key-value pairs as the keyword arguments to populate the dictionary. For example:
my_dictionary = dict(key1='value1', key2='value2', key3='value3')
Use the constructor when initializing a dictionary from various data sources. This method allows to dynamically create key-value pairs and convert various data types into dictionaries.
Method 3: Using Lists
The dict()
constructor, together with the zip()
function, enables merging two lists into key-value pairs. Provide the key values in one list and the values in a separate list. Then, combine the two using the zip()
function. For example:
keys = ['key1', 'key2', 'key3']
values = ['value1', 'value2', 'value3']
my_dictionary = dict(zip(keys, values))
The method is memory efficient and provides a straightforward way to combine two lists into a dictionary.
Method 4: Using Dictionary Comprehension
Dictionary comprehension is a memory-efficient way to initialize a Python dictionary. For example. provide two lists with key and value pairs, then combine them using dictionary comprehension:
keys = ['key1', 'key2', 'key3']
values = ['value1', 'value2', 'value3']
my_dictionary = {keys[i]:values[i] for i in range(len(keys))}
The code uses the range()
function and the list length to determine the number of iterations. Use this method when applying transformations to the keys or values during dictionary construction.
Method 5: Using Tuples
An alternative way to pass values into the dict()
constructor is to provide the key-value pairs as a list of tuples. For example:
my_dictionary = dict([('key1', 'value1'), ('key2', 'value2'), ('key3', 'value3')])
Use this method when working with a list of tuples to convert the data into a dictionary format easily. The constructor provides a convenient way to convert tuples into key-value pairs.
Method 6: Using the fromkeys()
The dict.fromkeys()
method creates a dictionary from a keys list using the same value for all keys. Provide the keys list and the default value to the method. For example:
keys = ['key1', 'key2', 'key3']
value = 'default_value'
my_dictionary = dict.fromkeys(keys, value)
Use the fromkeys()
method when different keys require the same default value during initialization.
Method 7: Using a Generator Expression
Generator expressions are similar to dictionary comprehensions. Both methods use iterables to generate a dictionary. An example generation expression looks like the following:
keys = ['key1', 'key2', 'key3']
values = ['value1', 'value2', 'value3']
my_dictionary = dict((key, value) for key, value in zip(keys,values))
Generator expressions are memory efficient and are suitable when working with large lists.
Initialize an Empty Dictionary
To initialize an empty dictionary, use one of the following two methods:
my_dictionary = {}
Or alternatively:
my_dictionary = dict()
Add items to the dictionary as needed using a method of your choice. For example, append a new key-value pair using the assignment operator to append the data to the initialized empty dictionary.
Conclusion
After reading this guide, you know several different ways to initialize a Python dictionary. Use a method best suited for your programming style and use case.