Strings are a textual immutable data type in Python. String appending (or concatenation) links multiple strings into one new object. Merging two or more strings into one is an elementary string operation with many use cases.
This article shows four different ways to append strings in Python.
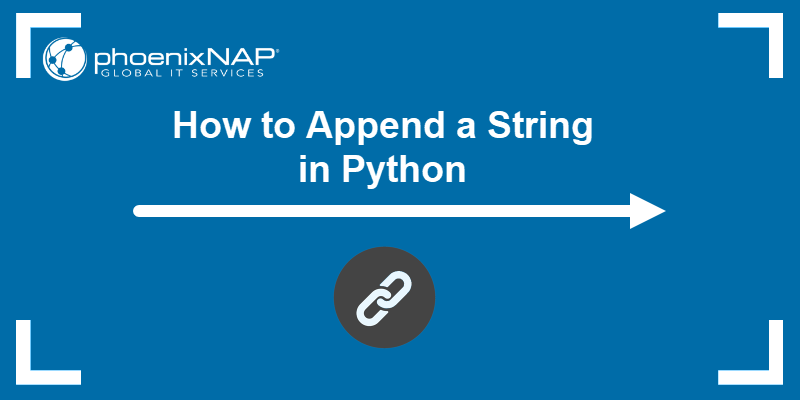
Prerequisites
- Python 3 installed.
- A text editor or IDE to write and edit code from the examples.
- Access to an IDE or terminal to run the code.
Different Methods to Append Strings in Python
Python offers several different methods to concatenate strings. The methods differ based on speed, readability, and maintainability. Choose a technique that best fits the use case in your program or script.
The sections below describe four different methods to append strings in Python through examples.
Method 1: Using + Operator
Use the plus (+
) operator to join two strings into one object. The syntax is:
<string 1> + <string 2>
The strings are either variables that store string types or string literals. For example:
str1 = "Hello"
str2 = "World"
print(str1+str2)
The operator concatenates the two strings and creates a new object. To add a space between the strings, append a string literal between two variables:
str1 = "Hello"
str2 = "World"
print(str1 + " " + str2)
Alternatively, add a space to the end of the first string or the beginning of the second string.
Use the (+
) operator to join two strings and generate an output quickly. This operator is slow for appending multiple strings compared to other methods.
Method 2: Using join()
The join()
method concatenates iterable objects (such as lists, tuples, or dictionaries) into one string. To use the method, call join()
on a string:
string.join(<iterable object>)
The string acts as a separator between the joined objects.
The following example shows the join() method in use:
str1 = "Hello"
str2 = "World"
print(" ".join([str1, str2]))
Calling the join()
method on a string with a space and providing a list of strings appends the strings and adds a separator between each element.
Use the join()
method when iterating through a list of strings, dictionaries, or other iterable objects.
Method 3: Using String format()
The format()
method creates a placeholder for objects and passes the provided values, effectively concatenating strings.
Define the placeholder using curly brackets ({}
) and pass the values into the format()
method:
"{} {}".format(<string 1>, <string 2>)
The provided values in the format()
method are inserted into placeholder spaces in the specified order. For example:
str1 = "Hello"
str2 = "World"
print("{} {}".format(str1, str2))
The output shows the concatenated string.
The format()
method allows controlling the string format and the appending order. For example, use indexing to reorder the string values:
str1 = "Hello"
str2 = "World"
print("{1} {0}".format(str1, str2))
The output shows the strings concatenate in reverse order.
Method 4: Using f-string
The f-string method uses literal string interpolation to format strings. The name comes from the syntax:
f"{<string 1>}{<string 2>}"
The preceding f
indicates the f-string format, while the curly braces ({}
) interpret provided variables. For example:
str1 = "Hello"
str2 = "World"
print(f"{str1} {str2}")
The method concatenates the two strings and adds a space between them.
Note: Learn how to repeat a string in Python.
Conclusion
You now know four different ways to append a string in Python. Refer to the provided examples to get started.
For operations with Python strings, check out Python substrings or string slicing.