Python is a versatile programming language for handling string data types and operations. String repetition is a common task in various programming and text manipulation tasks.
Whether you're repeating a string for testing purposes or require formatting texts, Python offers various methods to repeat a string.
This guide covers different approaches to string repetition in Python through practical examples.
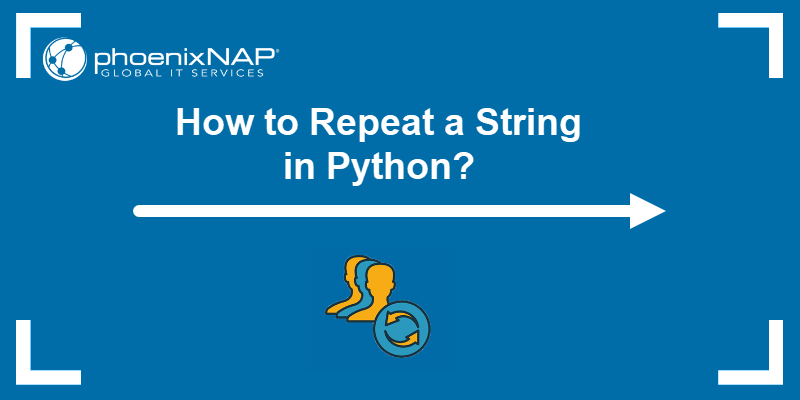
Prerequisites
- Python 3 installed and configured (check the Python version before starting).
- An IDE or text editor for Python.
- (Optional) Python modules, such as Itertool functions, NumPy, or Pandas.
Repeat a String via * Operator
The simplest way to repeat a string in Python is with the *
operator. Use the operator on a string to repeat the string a provided number of times.
The syntax for the operation is:
result = string * number
The code below demonstrates how to repeat the string "Hello, world!" five times:
result = "Hello, world!" * 5
print(result)
Alternatively, store the string and number in variables and change them as needed. For example:
my_string = "Hello, world!"
number = 5
result = my_string * number
print(result)
In both cases, the result is the string "Hello, world!" repeated five times.
Repeat a String via for Loop
Another built-in way to repeat a string in Python is using a for
loop and the range
function. Iterate over a provided range and append the string to a variable in each iteration.
The following code demonstrates the procedure to repeat a string five times:
result = ""
for _ in range(5):
result += "Hello, world!"
print(result)
The code does the following:
- Creates an empty placeholder string variable
result
. - Goes through five iterations using the
range(5)
function. - Appends the provided string each time to the
result
variable using the+=
operator. - Prints the result to the console.
Note: The underscore in the for
loop serves as a placeholder for the loop index variable.
To repeat a string and print on a new line each time, use the for
loop and print the string:
for _ in range(5):
print("Hello, world!")
The for
loop repeats the print
task five times and exits.
Repeat a String via itertools.repeat()
The itertools
library contains various iteration functionalities for Python. The itertools.repeat()
function creates an iterable object that repeats a string for a specified number of iterations. The syntax for the function is:
itertools.repeat(string, number)
The code below demonstrates how to use itertools.repeat()
to repeat a string in Python:
import itertools
result = "".join(itertools.repeat("Hello, world!", 5))
print(result)
The code uses the join()
method on an empty string to append the string iterable object to an empty string.
Alternatively, use the itertools.repeat()
function in a for
loop to repeat a string. For example:
import itertools
result = ""
for i in itertools.repeat("Hello, world!", 5):
result+=i
print(result)
The itertools.repeat()
function does not pre-generate the repeated values and is faster compared to the built-in range()
function.
Repeat a String via numpy.repeat()
The NumPy library has various numerical functionalities, including object repetition. The numpy.repeat()
function repeats a given object in a Python array. The syntax is:
numpy.repeat(string, number)
The operation result is an array with string elements. Join the array elements on an empty string to repeat a string using this method.
Note: This method requires installing NumPy.
The code below shows how to repeat a string via the numpy.repeat()
function:
import numpy as np
result_array = np.repeat("Hello, world!", 5)
result = ''.join(result_array)
print(result)
Use the numpy.repeat()
function when working with NumPy arrays or with array operations. When you are not working with arrays, use a simpler method to repeat a string.
Repeat a String for a Length With a User-Defined Function
If a code requires repeating different strings until a given string length, the most efficient approach is to create a custom function.
To create a function for repeating strings until a specified length, define the input and output variables. For example:
<b>input_string</b>
andlength
- The string to be repeated and the length of the repetition are the input values.result
- The resulting repetition is the output value.
The code below demonstrates how to perform string repetition until a given length:
def repeat_length(input_string, length):
number = int(length/len(input_string)+1)
result = input_string * number
print(result[:length])
repeat_length("Hello", 12)
repeat_length("World", 7)
The repeat_length()
function does the following:
- There are two user-input variables, the string (
input_string
) and the length of the final string (length
). - Line two determines the least number of repetitions necessary to get the correct number of characters.
- In line three, the function repeats the string for the calculated number of times using the
*
operator. - Lastly, the function prints the result up to the provided length using string slicing.
Call the function and provide a string and length to test the results.
Conclusion
After reading this guide, you know several ways to repeat a string in Python. As each method provides different benefits, knowing different ways to perform the same task is beneficial.
Next, learn how to use Python substrings to get parts of a string.