Reading from stdin (standard input) in Python is a common programming task that helps create interactive I/O programs. Stdin reads keyboard inputs from a user, files, or data streams.
Python offers several different methods to read from stdin depending on the type of problem.
This guide provides three methods to show how to read standard input from stdin in Python.
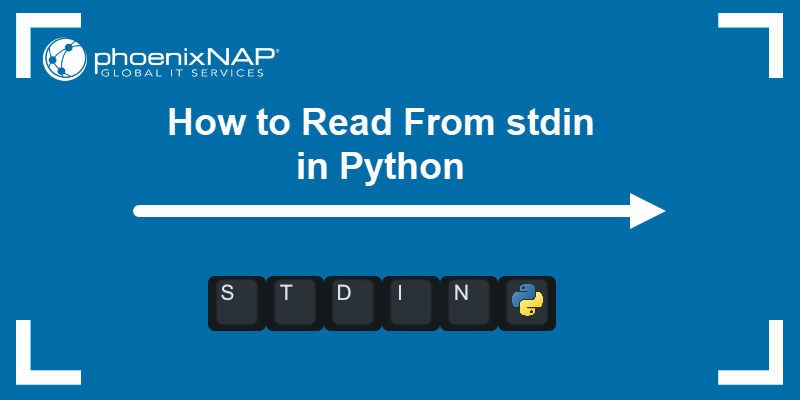
Prerequisites
- Python 3 installed and set up.
- Access to the command line/terminal.
- An IDE or code editor to write code.
Method 1: Read From stdin Using sys.stdin
The sys
module contains a stdin
method for reading from standard input. Use this method to fetch user input from the command line.
Import the sys
library and use the stdin
method in a for loop. See the example below for a demonstration:
import sys
print('Write a message and press Enter: ')
for line in sys.stdin:
line = line.rstrip()
print(f'Message from stdin: {line}')
break
The code does the following:
- Reads user input from the keyboard (
sys.stdin
). - Removes new lines from the user entry (
rstrtip()
). - Prints back the entered message by appending an f-string.
- Exits the for loop (
break
).
The program expects user input, prints the entered text stream, and returns to the command line.
Method 2: Read From stdin Using the input() function
The second way to read input from stdin in Python is with the built-in input()
function. Enter a descriptive message as a value, and save the output into a variable.
For example:
message = input('Enter a message:')
print(f'Your name is: {message}')
The program saves the input from stdin into a variable (name
) and returns the value.
Method 3: Read from stdin by Using fileinput.input()
The fileinput
Python library contains the input()
function, which allows reading file names from standard input.
The method allows passing file names as a function or command-line arguments. In both cases, the file's contents are read as input from stdin. Below is a demonstration of both use cases.
Files as Function Arguments
To pass file names as the fileinput.input()
arguments, the files must be located in the same directory as the script.
For example, if the current directory contains two files (file1.txt and file2.txt), use the following code to print the file contents line by line:
import fileinput
with fileinput.input(files=('file1.txt', 'file2.txt')) as f:
for line in f:
print(line)
The with
statement helps open a file stream, uses the file names as the function's input, then goes through each line in the file using a for
loop. As a result, the contents of the files print to the console.
Files as Command Line Arguments
An alternative way to read files is to provide the file names as command-line arguments. Use this method when reading different files each time.
The following code shows how to read files provided as command-line arguments:
import fileinput
for f in fileinput.input():
print(f)
Save the code and run with the following:
python3 test.py file1.txt file2.txt
The code goes through the list of files and prints the file contents to the console.
Conclusion
You now know three different methods to read input from stdin in Python. Standard input is an essential programming skill to get a user's input into a program.
Next, learn about file handling in Python or see how to take user input and create a calculator with Python.