Introduction
Python offers a range of functionalities to work with string data types. One such feature is the ability to extract substrings from a string.
Working with substrings is a valuable programming feature when dealing with textual data.
This guide teaches you how to substring a string in Python and the various use cases of substrings.
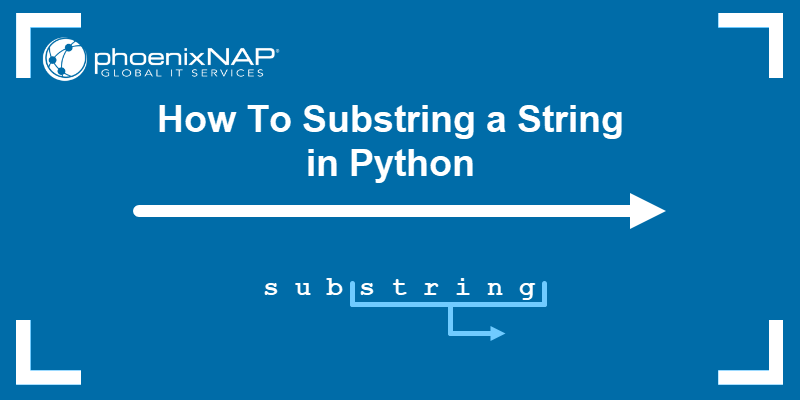
Prerequisites
- Python 3 installed (see how you can check the Python version).
- Access to a text editor or IDE.
- A way to run the code (an IDE or terminal).
What is a Substring in Python?
In Python, a substring is a character sequence inside a larger string, and every character has an index. The indexing can be:
- Positive (from the first character to the last)
- Negative (from the last character to the first)
The syntax for extracting substrings from strings is:
string[start:stop:step]
Python uses string slicing notation to work with substrings, where:
start
is the first character in the substring (default is the beginning).stop
is the first character excluded from the string (default is the end).step
is the distance between two characters (default is 1).
All three values can be positive or negative numbers. If left blank, the default values are assumed.
Alternatively, to divide a string into substrings based on a delimiter, use the split
method:
string.split("<delimiter>","<max splits>")
Without a provided delimiter, the method divides the string based on whitespaces. By default, the maximum number of splits is unlimited, which you can limit only to perform a maximum number of divisions.
Substring Operations in Python
Python offers different ways to create substrings depending on the task. The examples in the sections below demonstrate various problems and walk through the code syntax to explain the behavior.
Create a Substring
To create a substring using slicing, refer to the following code:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote[6:22])
The code prints a substring between two characters at the provided indexes.
Note: Learn how to perform string repetition in Python using several different methods.
Create a Substring From Beginning to Index
The following code shows how to make a substring from the beginning up to an index using slicing:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote[0:4])
print(quote[:4])
In both cases, the code prints a substring from the beginning. Use this method to fetch a substring before a specific character.
Create Substring from Index to End
To create a substring from a specific index to the end, see the following example:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote[43:])
print(quote[-8:])
In both cases, the code prints the end of a string after a specific character. Use negative indexing for substrings that are easier to count backward and regular indexing for substrings closer to the start.
Create Substrings Using Split
Use the split
method to create substrings based on a delimiter. For example:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote.split())
The output shows the code splits the given string by spaces and stores the result into a list of individual substrings.
Check if a Substring Exists
To see whether a substring exists within a string, use the in
operator and print the result:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print("Toto" in quote)
The code checks for the provided string and returns an appropriate message (True
or False
). The search performs an exact match and takes capitalization into account.
Count a Substring in a String
Use the count
method on a string and provide the substring to find the number of occurrences in a substring. For example:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote.count("a"))
The code counts the number of appearances of the letter "a
" in the string. Use this method to find the total number of instances of a substring.
Find the Index of the First Substring
To fetch the index of the first found substring, use the following example:
quote = "Toto, I have a feeling we're not in Kansas anymore."
print(quote.index("a"))
If the substring doesn't exist, the index
method returns an error.
An alternative way to find the index of the first substring is:
quote = "Toto, I have a feeling we're not in Kansas anymore."
string.find("a")
The find
method returns -1
if the substring is not found.
Note: To find the last occurring index, use the rindex
and rfind
methods.
Find All Indexes of a Substring
To find the indexes of all occurring substrings, use a for
loop and check with the startswith
string method. For example:
quote = "Toto, I have a feeling we're not in Kansas anymore."
for index in range(len(quote)):
if quote.startswith("a",index):
print(index)
The output prints all indexes of the substring in a new line.
Alternatively, use list comprehension to create a one-line solution:
quote = "Toto, I have a feeling we're not in Kansas anymore."
index_list = [index for index in range(len(quote)) if quote.startswith("a",index)]
print(index_list)
The resulting indexes are in a list. If there are no matches, the code returns an empty list.
Conclusion
You've learned how to create and work with substrings in Python. String slicing and various built-in methods simplify working with substrings. Knowing how to work with substrings is essential for tackling various text-based programming problems.