Introduction
Adding elements is a basic operation with Python arrays. There are multiple ways to define arrays in Python, such as with lists (an array-like data structure), the array module, or the NumPy library. Each method has different ways to add new elements.
Depending on the array type, there are different methods to insert elements.
This article shows how to add elements to Python arrays (lists, arrays, and NumPy arrays).
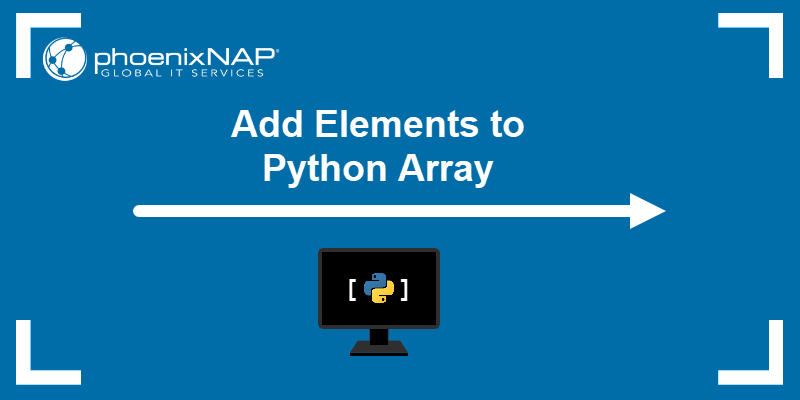
Prerequisites
- Python 3 installed.
- An IDE or text editor to write and edit code.
- An IDE or command line/terminal to run code.
- (optional) NumPy installed.
How to Add Elements to a Python Array?
Python lists act as array-like data structures that allow different element types. On the other hand, the array and NumPy libraries offer modules to create traditional arrays.
The methods to add an element to an array differ depending on the data type. Below is an overview of several possible methods.
Method 1: Adding to an Array in Python by Using Lists
If using lists as arrays, Python offers several built-in methods to add one or more elements to an existing list. The methods are:
- The (
+
) operator append()
extend()
insert()
Below is an overview of the three different methods with examples.
1. Add one or more elements to the end of a list with the (+
) operator. The operator adds a single value or another list (or iterable) to an existing list and updates the original variable.
To append one element to a list, see the following example:
l = [1, 2, 3]
print("List before adding:", l)
l = l + ["four"]
print("List after adding:", l)
Alternatively, append multiple elements:
l = [1, 2, 3]
print("List before adding:", l)
l = l + ["four", "five", "six"]
print("List after adding:", l)
The operator appends the elements to the end of the original list and increases the list length.
Note: Read our article and find out how to append a string in Python.
2. Add a single element to the end of a list with the built-in append()
method. The method does not support adding multiple values to a list.
For example, the code below shows how to create a list and append an element:
l = [1, 2, 3]
print("List before appending:", l)
l.append("four")
print("List after appending:", l)
The original list contains the new element at the end.
3. Add one or more elements to the end of a list with the built-in extend()
method. Provide a new list (or another iterable) as the method's parameter.
The example below shows how to add a new list to an existing one:
l = [1, 2, 3]
print("List before extending:", l)
l.extend(["four", "five", "six"])
print("List after extending:", l)
The old list extends with the new elements, which all append to the end of the list.
4. Add an element to an exact location in a list with the insert()
method. Provide the location index and the value to insert.
For example:
l = [1, 2, 3]
print("List before inserting:", l)
l.insert(1, 1.5)
print("List after inserting:", l)
The new element inserts at the provided index, while all the following values shift to the right by one index.
Method 2: Adding to an Array in Python by Using Array Module
The Python array module mimics traditional arrays, where all elements are restricted to the same data type. The module is similar to lists when it comes to adding new elements. The possible methods are:
+
operatorappend()
extend()
insert()
Below is a brief overview of each method using the array module.
1. Add one or more elements to an array using the +
operator. The element(s) append to the end.
The example below shows how to add to an array with the +
operator:
import array
a = array.array('i', [1,2,3])
print("Array before adding:", a)
b = array.array('i', [4,5,6])
a = a + b
print("Array after adding", a)
Both arrays use the same type code ('i'
for unsigned integer). The +
operator appends the new set of numbers to the existing one.
2. Add a single element to an array with the append()
method. The method appends a new value to the end, which must match the array element type.
See the following example to use the append()
function to add a new element to an array:
import array
a = array.array('i', [1,2,3])
print("Array before appending:", a)
a.append(4)
print("Array after appending:", a)
The method extends the existing list with a new element, which matches the array type code.
3. Add one or more elements to an array end with the extend()
method. Provide the elements in an iterable data type. The items in the iterable must match the array's data type.
For example, add multiple elements to an array with a Python list:
import array
a = array.array('i', [1,2,3])
print("Array before extending:", a)
a.extend([4,5,6])
print("Array after extending:", a)
The extend()
method links the list to the array.
4. Add an element to a specific place in an array with the insert()
method. Set the element index and provide the element value to insert the value.
For example:
import array
a = array.array('i', [1,2,3])
print("Array before inserting:", a)
a.insert(1,0)
print("Array after inserting:", a)
The value 0
inserts at index 1
, pushing all the following elements to the right.
Method 3: Adding to NumPy Array
The NumPy library contains an array type suitable for numerical calculations. Unlike lists and arrays, fewer ways exist to add elements to a NumPy array. The methods are:
append()
insert()
Both methods work as functions that require the array and appended values.
Below are explanations and examples of both methods for inserting elements into a NumPy array.
1. Use the append()
method to add one or more elements to a copy of a NumPy array. Provide the original variable and add the new values to an iterable.
For example:
import numpy as np
n = np.array([1, 2, 3])
print("NumPy array before appending:", n)
n = np.append(n, [4, 5, 6])
print("NumPy array after appending:", n)
The append()
method adds a list to the NumPy array and overwrites the old variable with new values.
2. Use the insert()
method to add one element at a specific location to a copy of a NumPy array. Provide the NumPy array, index, and value to add a new element.
For example:
import numpy as np
n = np.array([1,2,3])
print("NumPy array before inserting:", n)
n = np.insert(n,1,0)
print("NumPy array after inserting:", n)
The method inserts a new value (0
) at index 1
and pushes all following values by one to the right.
Conclusion
After reviewing the methods from this guide, you know how to add an element to an array.
Whether you're working with lists, arrays, or NumPy arrays, Python offers several methods for each data type to insert new values.