Reading files is a common task in Python, whether you're parsing data, loading configuration files, or viewing log files.
There are multiple ways to read files in Python, and each method is suitable for a different scenario.
This guide will show common ways to read a file in Python with examples.
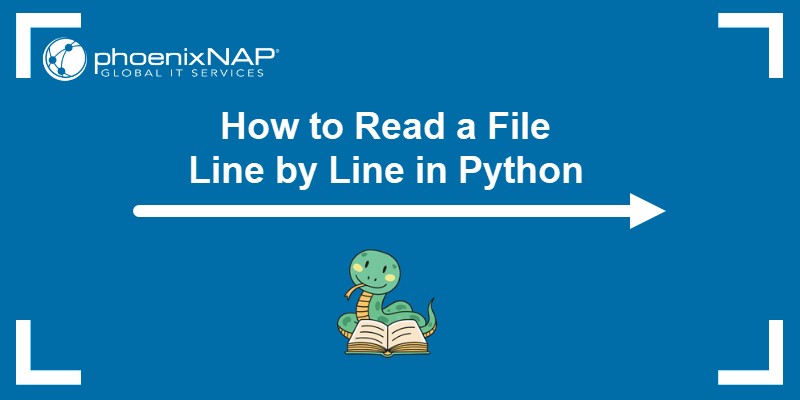
Prerequisites
- Python 3 installed (we're using 3.12).
- Access to a Python IDE or editor.
- A text file to test the code.
Note: To install Python 3, try one of our guides for your operating system:
Read a File in Python Using for Loop
A for loop is a memory-efficient way to read a file line by line. This approach iterates over a file object directly.
For example:
my_file = 'sample.txt'
with open(my_file, 'r') as file:
for line in file:
print(line.strip())
In most cases, the loop approach is sufficient. It's simple, efficient, and easy to read.
Note: The open()
method is essential when working with files in Python. For more information, read our in-depth guide on file handling in Python.
Read a File in Python Using readline() Method
The readline()
method reads a line from a file each time it is called. This method offers better control over processing each line and allows conditional statements between reads.
To read a file line by line using the readline()
method, try the following code:
my_file = 'sample.txt'
with open(my_file, 'r') as file:
line = file.readline()
while line:
print(line.strip())
line = file.readline()
Use this method to skip specific lines in a file, stop reading the file under some condition, or address reading errors.
Read a File in Python Using readlines() Method
The readlines()
method stores a file's lines into a list (an array-like Python data type). The method is helpful for accessing specific lines in a file or batch-processing lines after loading a file.
The code below demonstrates how the method works:
my_file = 'sample.txt'
with open(my_file, 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
The readlines()
method is unsuitable for large files since it loads the whole file into memory before processing.
Read a File in Python Using pathlib and splitlines()
The pathlib
module enables reading a file as a single string. The splitlines()
method helps divide the string into lines.
The example below shows how to use the module and method together:
from pathlib import Path
my_file = Path('sample.txt')
lines = my_file.read_text().splitlines()
for line in lines:
print(line)
Use this approach if pathlib
is already in your workflow. Avoid it if working with large files, as it first loads the entire file into memory.
Read a File in Python Using fileinput Module
The fileinput
module allows reading from standard input or files. The flexible module allows working with multiple files or using input redirection.
For example:
import fileinput
my_file = 'sample.txt'
for line in fileinput.input(files=my_file):
print(line.strip())
The module is practical when creating automation scripts or command-line tools.
Read a File Using Generator Function
A generator function is a modular approach for reading lines from a file. It's also flexible, since you can create a custom function that integrates into your workflow.
See the example below:
def read_line(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
my_file = 'sample.txt'
for line in read_line(my_file):
print(line)
A generator function is ideal for re-usability and iterative processing.
Conclusion
This guide showed several ways to read files line by line in Python. Each method has specific advantages and is better suited for different needs.
Next, see how to read files line by line in Bash.