Introduction
Object-oriented databases (OODB) are database management systems with unique functionalities. This specialized database type adds database functionality to object programming languages, creating a more manageable codebase.
This article gives an in-depth overview of object-oriented databases with examples.
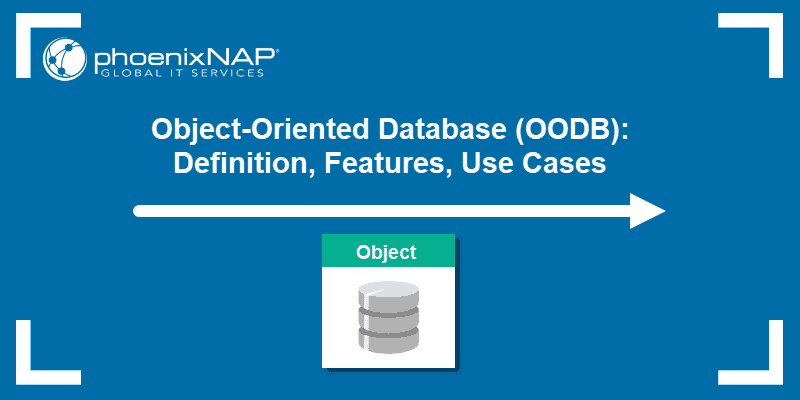
What Is an Object-Oriented Database (OODB)?
An object-oriented database (OODB) is a database that combines object-oriented programming concepts with relational database principles. It is managed by an object-oriented database management system (OODBMS). OODBs contain the following elements:
- Objects. The basic building block and an instance of a class. The type is either built-in or user-defined.
- Classes. A schema or blueprint that defines object structure and behavior.
- Methods. A blueprint that defines the behavior of a class.
- Pointers. An entity that helps access elements of an object database. They also help establish relationships between objects.
The main feature of objects is the possibility of user-constructed types. An object created in a programming language (such as Java) saves into an OODB as is. All the information about an object is in a single package instead of spread across multiple tables.
Object-Oriented Database (OODB) vs. Other Types of Databases
Object-oriented databases significantly differ from other database types in their design and functionality. Knowing these differences can help determine when using an OODB is the best choice. Below is a brief comparison between OODB and other types of databases.
OODB vs. Relational Databases
The main difference between the two is how the data is structured:
- Relational databases. Data resides in structured tables with rows and columns. It is queried using SQL, focusing mainly on data consistency and normalization.
- OODB. Data is stored as a complete and complex object. It supports complex data types and inheritance. The main focus is to minimize the difference between a database structure and a programming language.
OODB vs. Document-Oriented Databases (NoSQL)
A notable difference is in their applicability:
- Document-oriented databases (NoSQL). Data is unstructured or semi-structured. Caters to various data models, with a focus on scalability and flexibility.
- OODB. Manages objects with built-in relationships and behaviors. Ideal for complex data structures or for integration with object-oriented programming.
OODB vs. Key-Value Databases
The two database types differ in complexity:
- Key-value databases. A simple structure with key-value pairs. Ideal for lookup tables and data caching, but there is no support for complex relationships.
- OODB. Supports complex relationships, inheritance, and encapsulation.
OODB vs. Graph Databases
Relationships are a key difference between these two database types:
- Graph databases. Structured for highly connected data. Uses edges and nodes to represent relationships between entities.
- OODB. Focuses on objects and their behavior while supporting relationships, though not as interconnected as graph databases.
Object-Oriented Programming Concepts in Database Management
Object-oriented databases closely relate to object-oriented programming (OOP) concepts. The four main features of OOP are:
- Polymorphism.
- Inheritance.
- Encapsulation.
- Abstraction.
These four attributes describe the main characteristics of object-oriented management systems in general. The sections below explain each of these concepts in greater depth.
Polymorphism
Polymorphism is the capability of an object to take multiple forms. This ability allows the same program code to work with different data types.
To illustrate, a Vehicle
class can be defined to have a method called brake()
. A Car
and a Bike
can both inherit from the class and implement their version of the brake()
method. The same method is applied for different behaviors, resulting in polymorphism.
Inheritance
Inheritance creates a hierarchical relationship between related classes while making parts of code reusable. Defining new types inherits all the existing class fields and methods plus further extends them. The existing class is the parent class, while the child class extends the parent.
For example, a parent class called Vehicle
can have child classes such as Car
and Bike
. Both child classes inherit information from the parent class. They also extend the parent class with new information depending on the methods defined for each vehicle type.
Encapsulation
Encapsulation allows grouping variables and methods into a single object to create access protection. This process hides information and details of how an object works from the rest of the code and results in data and function security.
Classes interact with each other through interface methods without the need to know how particular methods work.
For example, a Car
class can have properties such as color
, make
, and model
and methods such as changeColor()
. You can change the color of a car through a method, yet the model
and make
are not accessible. Encapsulation bundles all the car information into one entity, where some elements are modifiable while some are not.
Abstraction
Abstraction is the process of focusing on the essential characteristics to provide functionality. The process selects vital information while unnecessary details stay hidden. Abstraction helps reduce data complexity and simplifies code reusability.
For example, when a web browser connects to the internet, it doesn't need to know the specific connection details. Whether the connection is established through Wi-Fi or Ethernet is irrelevant. The specific connection type is hidden from the browser to create an abstraction, whereas the various types of connections represent different implementations of the abstraction.
Note: For more information about object-oriented programming principles, see our in-depth analysis of SOLID design principles.
Features of Object-Oriented Databases
OODB has different features and implementations. Most contain the attributes described in the table below:
Feature | Description |
---|---|
Query Language | Finds objects and retrieve data from the database. |
Transparent Persistence | Allows accessing and using data with an object-oriented programming language without special handling. |
ACID Transactions | Ensures that ACID transactions, and guarantees all transactions are completed without conflicting changes. |
Database Caching | Creates a partial replica of the database in memory. Allows faster access to a database without reading from disk. |
Recovery | Provides disaster recovery mechanisms in case of application or system failure. |
Object-Oriented Database Management Systems (OODBMS)
Object-oriented database management systems (OODBMS) integrate object-oriented principles into a database technology. It enables developers to use object-oriented languages to manage complex data models intuitively.
The sections below show several notable examples of OODBMS. Each caters to different platform and application requirements.
GemStone/S
GemStone/S is an object database system based on Smalltalk, an object-oriented programming language influenced by Java. Developers who write applications in Smalltalk can quickly adapt to this database, although the language is uncommon. GemStone/S integrates seamlessly with existing applications written in this language, improving speed and productivity.
This system is best for high-availability projects. There are multiple options for licensing depending on the project size. The database server is available for various operating systems, including Linux, Windows, macOS, Solaris, AIX, and Raspberry Pi OS.
Note: Learn all about how database servers work to find out how they can be an asset to your system.
ObjectDB
ObjectDB is a NoSQL object database for the Java programming language. Compared to other NoSQL databases, ObjectDB is ACID compliant. ObjectDB does not provide an API and requires using one of the two built-in Java database APIs:
- JPA with JPA Query Language (JPQL) based on Java syntax.
- JDO with JDO Query Language (JDQL) based on SQL syntax.
ObjectDB includes all basic data types in Java, user-defined classes, and standard Java collections. Every object has a unique ID. The maximum database size (128 TB) limits the number of elements. ObjectDB is available cross-platform, and its benchmark performance is exceptional.
ObjectDatabase++
ObjectDatabase++ is a real-time embedded object database designed for server-side applications. The required external maintenance is minimal.
ObjectDatabase++ supports:
- Multi-process with multi-threaded server applications.
- Full transaction control.
- Real-time recovery.
- C++-related languages, VB.NET, and C#.
The object database is based on C++. One of the main features is advanced auto-recovery from system crashes without compromising the database integrity.
Note: Learn more about big data processing by referring to our post Big Data Servers Explained.
IgniteTech
IgniteTech offers ObjectStore, an enterprise-grade embedded OODBMS that integrates with C++ or Java and provides memory persistence. Its responsiveness allows developers to build distributed cross-platform applications on-premises or in the cloud.
The main feature is cloud scalability, which enables achieving a distributed database. ObjectStore simplifies the data creation and exchange process seamlessly.
ZODB
ZODB (Zope Object Database) is an OODB based on Python. Its design is simple and aims to integrate with Python applications seamlessly. The database requires minimal code changes and provides transparent persistence for Python objects.
ZODB supports ACID transactions, object versioning, and storing large datasets. It fully integrates with Python and is an obvious choice for developers requiring a flexible object database.
Perst
Perst is McObject's lightweight OODB that integrates with Java and .NET applications. Its small footprint is ideal for embedded systems and mobile applications.
The database supports transparent persistence for Java and .NET objects. It also includes XML support and full-text search capabilities. A key feature is its efficient approach to storing time-series data, but developers can also implement custom data structures to ensure optimal performance for their specific use case.
Benefits of Object-Oriented Databases
The main advantages of OODBs are:
- Complex data and a wider variety of data types compared to MySQL data types.
- Easy to save and retrieve data quickly.
- Seamless integration with object-oriented programming languages.
- Easier to model advanced real-world problems.
- Extensible with custom data types.
Challenges of Object-Oriented Databases
Some disadvantages of OODBs include:
- It is not as widely adopted as relational databases.
- No universal data model. Lacks theoretical foundations and standards.
- Does not support views.
- High complexity, which may lead to performance issues.
- An adequate security mechanism and access rights to objects do not exist.
Object-Orient Databases: Use Cases
Object-oriented databases are beneficial in situations where there are complex data structures and relationships. Below are common use cases for OODB:
- Multimedia applications. OODBs store and handle multimedia objects efficiently. Inheritance and encapsulation are advantages when working with this content type.
- CAD/CAM systems. Engineering, automotive, and aerospace applications use CAD/CAM data. This data has highly interconnected elements and is best modeled as database objects.
- Geographic information systems (GIS). Representing and managing spatial data, such as maps and terrain information, requires complex relationships and properties. These features, such as coordinates and topographical relationships, are best suited for object-oriented structures.
- Telecommunications. OODBs efficiently handle hierarchical and interconnected data structures typical for telecommunication networks. The data includes network configurations, logs, and user preferences.
- Real-time systems. OODBs enable quick updates and retrieval of complex data essential for real-time systems. These technologies are found in industrial automation, robotics, and embedded applications.
Conclusion
This guide explained object-oriented databases in great detail. OODBs provide a modern approach to database modeling, mirroring the objects used in object-oriented programming.
To learn more about database technologies, see our blog article What is database as a service (DBaaS).