Encapsulation is a fundamental concept in object-oriented programming that involves bundling data and methods that operate on that data within a single unit, typically a class.
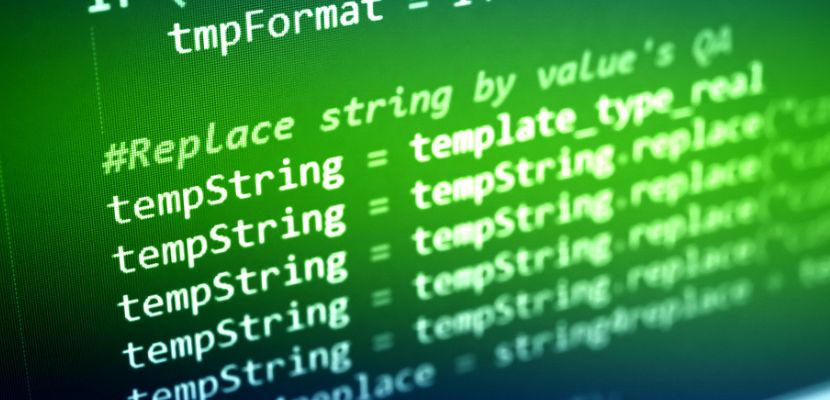
What Is Encapsulation in Object-Oriented Programming?
Encapsulation in object-oriented programming is the practice of bundling the data (attributes) and the methods (functions) that operate on that data into a single unit, or class. It restricts direct access to some of an object's components, protecting the integrity of the data by preventing outside interference and misuse. This is achieved by defining the access level of class membersโsuch as private, protected, or publicโcontrolling how the internal state of an object can be accessed or modified.
Encapsulation promotes modularity, reduces complexity, and enhances code maintainability by keeping the implementation details hidden and exposing only what is necessary.
Access Modifiers
Access modifiers in object-oriented programming are keywords that define the visibility and accessibility of classes, methods, and variables. They control how different parts of a program can interact with each other, ensuring that certain elements are only accessible where they are intended to be used.
The most common access modifiers are public, private, and protected:
- Public allows access from any part of the program.
- Private restricts access to the class in which the member is defined.
- Protected permits access within the class and its subclasses.
By using access modifiers, developers can enforce encapsulation, protect data integrity, and create clear boundaries between different components of the code.
Encapsulation vs. Decapsulation
Encapsulation and decapsulation are opposing processes in networking and data transmission. Encapsulation involves wrapping data with the necessary protocol information as it moves down the layers of the OSI model, such as adding headers and trailers to form a complete packet that can be transmitted over a network. This ensures that the data is properly formatted and routed to its destination.
Decapsulation, on the other hand, is the reverse process, where the receiving device strips away these added layers to extract the original data as it moves up the OSI layers. While encapsulation prepares data for transmission, decapsulation is crucial for correctly interpreting and using the received data.
Encapsulation in Java
Encapsulation is essential in Java because it provides control over the access and modification of an object's internal state, promoting data integrity and security. By restricting direct access to the fields of a class and exposing only specific methods (getters and setters) for interaction, encapsulation prevents external code from altering the object's data in unpredictable or unauthorized ways.
Encapsulation not only safeguards the data but also allows for greater flexibility in maintaining and updating the code. Changes to the internal implementation can be made without affecting the external interface, ensuring that other parts of the program continue to function correctly. Encapsulation also enhances code readability and maintainability by clearly defining how data should be accessed and manipulated, making the codebase more robust and easier to manage.
Data Hiding vs. Encapsulation in Java
Data hiding is the practice of restricting access to the internal data of a class, typically by declaring fields as private and using access modifiers to control visibility. This ensures that the internal state of an object is shielded from direct external modification, thereby protecting the integrity of the data.
Encapsulation, on the other hand, is a broader concept that not only involves data hiding but also includes bundling the data with the methods that operate on it within a single class. While data hiding focuses on safeguarding the data, encapsulation organizes and manages how data is accessed and manipulated, providing a structured interface to the outside world.
In essence, data hiding is a technique used to achieve encapsulation, which is a fundamental principle of object-oriented design.