Introduction
Docker supports environment variables as a practical way of externalizing a containerized app configuration. When included in a Docker image, environment variables become available to all deployed containers.
This article shows how to set Docker environment variables when creating Docker images. It also provides instructions for overriding the default variable values in existing images.
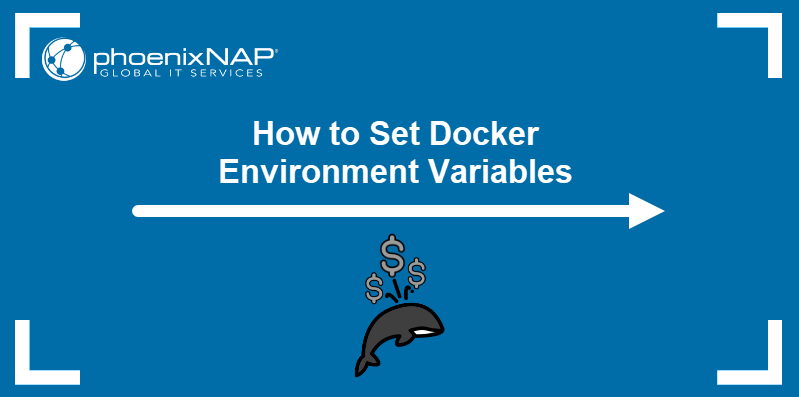
Prerequisites
- Docker installed (read our installation manuals for Ubuntu and Rocky Linux).
- Administrative access to the system or non-root access to Docker enabled.
- Docker Compose installed (where applicable).
What Are Docker Environment Variables?
Environment variables are dynamic key-value pairs that store information about the environment in which a process is running. In Docker, they can provide configuration settings and context to containerized apps.
For example, an environment variable can store a database URL that tells an app where to connect with a PostgreSQL instance. If this variable is stored as part of a Docker image, the containerized app can use it to establish the connection with the database each time a container is created.
Types of Docker Environment Variables
Docker supports two environment variable types:
ARG
variables usually store important high-level configuration parameters, such as the version of the OS or a library. They are build-time variables, i.e., their only purpose is to assist in building a Docker image. Containers do not inheritARG
variables from images. However, users can later retrieveARG
values from an image with thedocker history
command.ENV
variables store values such as secrets, API keys, and database URLs. UnlikeARG
s, they persist inside the image and its containers. Users can overrideENV
values in the command line or provide new values in a .env file.
How to Set Docker Environment Variables
The following sections describe how to define variables and assign them default and modified values. The methods involve Dockerfile, Docker Compose, and Docker CLI commands.
Via Dockerfile
Dockerfile allows users to define ARG
and ENV
variables using a simple syntax. Create an ARG
variable by adding the following line to the Dockerfile:
ARG [key]
For ENV
variables, use the ENV
specifier:
ENV [key]=[value]
Note: ARG
variables are placeholders that enable passing values to a Dockerfile at build time. While uncommon, assigning a default value to the ARG
variable in a Dockerfile is possible using the ARG [key]=[value]
syntax.
For example, to add one ARG
and one ENV
variable to a Dockerfile using the alpine:latest template, enter the following:
FROM alpine:latest
ARG test_one
ENV test_two='test_value'
Via docker build Command
The docker build
command creates images using instructions provided in Dockerfiles. It also features the --build-arg
option, which allows users to pass the value to the previously defined ARG
variable. The syntax is as follows:
docker build -t [image-name] --build-arg [arg-variable]=[value] .
The example below assigns the test_one
variable with new_value
.
docker build -t test_image --build-arg test_one='new_value' .
Use --build-arg with ENV variables
By default, the --build-arg
option modifies ARG
values. To use the option with ENV
variables:
1. In a Dockerfile, assign the ARG
variable's name as the value of ENV
. For example:
ARG test_one
ENV test_two=$test_one
2. Build the image. Use the --build-arg
option to pass a value to ARG
:
docker build -t test --build-arg test_one='example' .
Docker processes the ARG
value and assigns the value 'example' to ENV
.
Via docker run Command
The docker run
command allows users to create environment variables and pass them to a container at runtime. To create a variable with docker run
, use the -e
option:
docker run -e [key]=[value] [image]
For example, to add a variable named test
with the value true
to the container made using the test_image image, enter the following:
docker run -e test='true' test_image
Via environment Attribute
Docker Compose allows you to specify environment variables directly by using the environment
attribute. Below is the YAML syntax for this attribute:
...
environment:
- [key]=[value]
- [key2]=[value2]
...
For example, to create in-line definitions for the variables listed in the .env file above, type the following:
version: '3.7'
services:
test-app:
image: example/test-app:0.2
environment:
- DATABASE_NAME='test_database'
- DATABASE_USER='pnap'
- DATABASE_PASSWORD='st40xDN68d3'
- DATABASE_HOST=test-server.example.com
- DATABASE_PORT=3306
Via External .env File
A .env file is a text file storing key-value pairs that Docker can interpret and use as environment variables. Each line in the file is a single key-value pair:
[key]=[value]
The example below shows a .env file that defines five variables.
If a .env file is placed in the project's root directory, Docker Compose will automatically load the variables defined in it. If the file has any other name or is located elsewhere, use the env_file
attribute to tell Docker Compose where to look. For example, to load variables from a file named test_vars located in /home/test/docker-project, use env_file
as shown below:
version: '3.7'
services:
test-app:
image: example/test-app:0.2
env_file:
- /home/test/docker-project/test_vars
For deployments that do not use Docker Compose, use the --env-file
flag with a docker run command to specify the path to the file. Using the example above, the command is:
docker run --env-file /home/test/docker-project/test_vars example/test-app:0.2
Via Shell Substitution
Docker loads variables defined in Dockerfile and compose.yml each time it creates a new container. To temporarily change one or more variables, use shell substitution and specify the variables with the docker-compose up
command.
For example, to temporarily log in to a database using different user credentials, type the following command:
docker-compose up -e DATABASE_USER='test' DATABASE_PASSWORD='testpass'
Based on this command, Docker creates a container using the new DATABASE_USER
and DATABASE_PASSWORD
values, overriding those defined in the Docker Compose file. The change persists only during the lifetime of the specific container.
How to View Docker Environment Variables
Users can view environment variables in Docker by executing the docker inspect
command on a container or by using docker exec
to run the env
command from within a container. Read the sections below to learn both methods.
Via docker inspect Command
The docker inspect
command allows users to see the configuration parameters of a container. Inspect the container by typing:
docker container inspect [container-name-or-id]
The Config
section of the output contains a list of environment variables in the container.
Via docker exec Command
The docker exec
command can execute the env
command within a running container and show a list of environment variables. The syntax is as follows:
docker exec [container_id] env
How to Override Docker Environment Variables
Users override ENV
variable defaults in multiple ways. In order of precedence, the application considers the values set by:
- The containerized application itself.
- The
-e
command-line argument of thedocker run
command. - The .env file provided through the
docker run
--env-file
option. - The Dockerfile.
Override Single ENV Variable
Use the -e
option with docker run
to override a single defined ENV
variable when creating a container.
docker run --name [container-name] -e [variable-name]='[new-value]' [image-name]
The example below changes the value of test
to runtime_value
while creating the test_container
from test_image
:
docker run --name test_container -e test='runtime_value' test_image
Override Multiple ENV Variables
Provide a set of variable values on runtime by creating an .env file that contains the relevant key-value pairs.
1. Create the file with a text editor:
nano [env-filename]
2. Populate the file with key-value pairs:
[variable1]=[value1]
[variable2]=[value2]
[variable3]=[value3]
...
3. Save the file and exit.
4. Pass the values from the file with the --env-file
option.
docker run --name [container-name] --env-file [path-to-env-file] [image-name]
Note: Containers are an essential part of every Kubernetes deployment. To improve container management efficiency and quickly deploy a production-ready Kubernetes environment, use Rancher on Bare Metal Cloud.
Conclusion
After reading this tutorial, you should know how to define and set ARG
and ENV
environmental variables. The article also showed how to override their values using Docker CLI and Docker Compose.
Next, learn how to mount NFS Docker volumes.