Introduction
Dependency injection is a design convention that resolves code dependencies using the inversion of control (IoC) principle. The pattern creates seamless communication between adaptable software components.
A critical challenge in software development is managing and understanding dependency injection. A developer applying the design pattern produces readable, efficient, and flexible code.
This article will teach you about dependency injection, what it is, how it works, and the pros and cons.
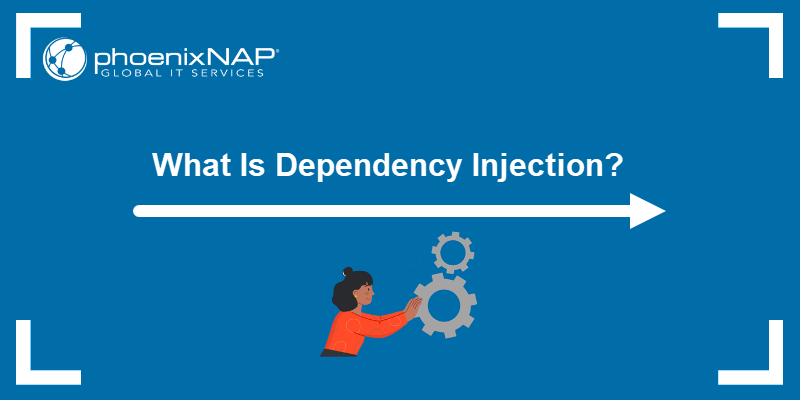
What Is Dependency Injection?
Dependency injection (DI) is a programming method that separates component creation and usage. The design pattern appears in object-oriented programming and adheres to the SOLID principles (specifically the S and the D). Similar ideas also apply to other programming paradigms, such as procedural or functional programming.
The term consists of two keywords, which help demystify the concept:
- Dependencies. Code components rely on many objects and services (dependencies) to carry out their tasks. Different dependencies exist, such as outside resources, other objects, or services.
- Injection. Components do not make or link dependencies internally in DI. Instead, the technique provides (injects) dependencies from the outside. This approach creates a separation between components and dependencies.
Dependencies come in various shapes and forms. They are essential for a component to work but are not part of the component itself. APIs, libraries, databases, and other components can all act as dependencies.
Dependency injection solves the dependency management challenge. By separating dependency management from a component, an external source creates and manages the dependencies.
Separation (or decoupling) reduces the number of connections between components and modules. Fewer connections result in simplified maintenance and flexibility. As a result, code becomes reusable because components are independent and applicable to various contexts. Teams do not require constant coordination and can develop modules in parallel.
Why Use Dependency Injection?
There are several key reasons to use dependency injection. Some of the main benefits include:
- Readability. DI promotes division between a component's logic and its dependencies. Code is easier to read and better organized.
- Reusability. Components with injected dependencies are easy to reuse for different projects due to being independent. DI provides a templating approach to coding, reducing repeated code blocks and rewriting across different projects.
- Testability. Dependency injection simplifies writing unit tests for individual components. Regular testing leads to dependable code with fewer bugs.
- Maintainability. Due to fewer connections between components and modules, changing or improving individual code parts does not affect other parts of the code.
Dependency Injection Types
Developers use various techniques to implement dependency injection. Choose the approach that best suits the requirements, programming language, and use case. Below is a brief overview and example of some dependency injection types.
Constructor Injection
Constructor injection is a dependency injection type that injects dependencies through a constructor. When an instance of a class or object is created, the constructor provides the dependencies during creation. As a result, the class or object runs correctly and immediately has all the necessary dependencies.
Use constructor injection when the dependencies are required for a class or object to function. The dependencies are injected during class or object instantiation and make dependencies immediately available to a class or object instance.
Setter Injection
Setter injection is a dependency injection that provides dependencies through setter methods or class properties. A developer updates the dependencies manually after object or class instantiation. As a result, the object receives appropriate dependencies when the setter methods are called.
Use setter injection when a class or object functions without mandatory dependencies. In this case, the dependencies are optional during instantiation and can be injected after runtime. This approach allows modifying dependencies dynamically after object creation.
Method Injection
Method injection (or parameter injection) is a dependency injection type that provides dependencies as method parameters. The class or object expects dependencies as arguments when calling a method.
Use method injection for fine-grained dependency injection based on individual methods. Method injection achieves the highest decoupling degree and is useful when a class has multiple methods that do not require the same dependencies.
How Does Dependency Injection Work?
The dependency injection process uses four main components (roles):
1. Service. A component that provides a function or service. Other components depend on the provided service.
2. Client. A component or class that depends on the service to perform tasks. The client does not create or manage the service; it relies on a DI process to provide the service.
3. Injector. Creates and manages service instances and injects them into clients. The injector resolves all dependencies and connects components as required.
4. Interface. The interface (or contract) defines methods or properties a service implements. The abstraction is an agreement between the service and the client, ensuring the service addresses all rules and expectations.
The dependency injection process goes through the following steps:
- Defining dependencies. Determine resources that classes and components need to function.
- Defining interfaces (contracts). Create an interface for each dependency. Provide the methods or properties that serve as contract rules.
- Injecting dependencies. Choose a mechanism to inject dependencies into classes or components. Depending on the mechanism, this step happens manually, with a DI container or a framework.
- Using dependencies. Classes and components use the dependencies by interacting with the defined contracts.
Dependency Injection Advantages and Disadvantages
There are both advantages and disadvantages when implementing dependency injection. Although the approach improves code maintainability, there's a steep learning curve and added code complexity.
Below is a brief overview of DI's main advantages and disadvantages.
Advantages
The main advantages of dependency injection are:
- Logical separation. Loose coupling between components and dependencies makes code easier to read and maintain. Classes and objects do not handle dependency instantiation or management.
- Improved testing. DI simplifies unit testing. Creating mock dependencies or testing individual components is simplified.
- Flexible dependency management. Separating dependencies and components provides flexibility. Different dependencies can be injected, which creates an easily adaptable environment.
- Reusable components. Due to loose coupling between components and dependencies, the code is reusable in different contexts and environments.
- Simpler maintenance. Upgrading libraries or components does not affect the underlying dependent class.
- Concurrent development. Developers can work on modules and dependencies in parallel while adhering to the defined contracts.
Disadvantages
The disadvantages of dependency injection are:
- Complexity. When handling many dependencies or complex configurations, DI additionally increases code complexity.
- Learning curve. The concepts behind DI and when to apply them take time to grasp fully. Initial project development slows down due to the learning curve.
- Overhead. DI is not suitable for smaller projects, as it adds redundant overhead.
- Runtime errors. Dependencies that are not carefully injected result in runtime errors. Troubleshooting is challenging, especially in complex environments.
Conclusion
After reading this guide, you know what dependency injection is and how it can improve code maintainability.
Next, learn more about software testing methodologies and their role in creating high-quality software.