The echo
command in Windows PowerShell is used to print text to the console. The command is an alias for the Write-Output
cmdlet.
This article helps you understand how to use echo in PowerShell and learn the differences between echo
, Write-Output
, and Write-Host
.
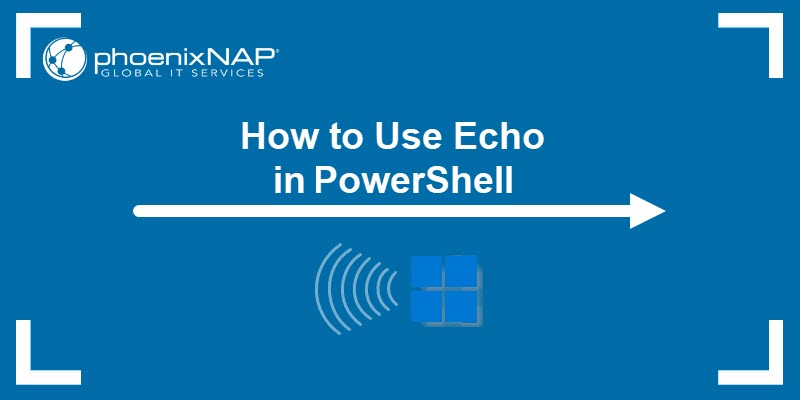
Write-Output and Echo in PowerShell
The echo utility is a Windows legacy command from the DOS operating system, still supported in Windows for compatibility reasons.
The main echo function in the Command Prompt (CMD) is to print a message to the console as long as the message is in quotation marks. The echo
syntax is:
echo ["message"]
For instance, run the following command:
echo "This is a sentence."
The output prints the This is a sentence message.
Note: Learn how to use the echo command in Linux.
PowerShell is a more powerful tool than CMD and uses cmdlets to perform tasks. One cmdlet is Write-Output
, which displays output in the console. The echo
command is used as an alias for Write-Output
, so the two commands are interchangeable in many cases.
Both echo
and Write-Output
have numerous features:
- Text printing.
- Object printing.
- Formatting the output using the
-Format
cmdlets. - Specifying the output encoding.
- Redirecting the output to a file.
- Parameters that allow users to customize the output.
Example of echo in PowerShell
Both echo
and Write-Output
have the same functionality and flexibility. Still, many users prefer Write-Output
for better clarity and readability in scripts with advanced functions.
The basic echo
output in PowerShell is similar to the one in CMD:
echo "This is another sentence."
The command prints the text in the output. However, echo
offers additional options regarding formatting the output and working with different parameters. For example, set the parameter $name
:
$name = "Sara"
Next, run echo
on the variable:
echo $name
The output prints the content of the variable.
echo
also supports formatting the output by piping it to a Format-
cmdlet.
Available cmdlets are:
Format-Custom
. Customizes the output with different views.Format-Hex
. Shows data in hexadecimal format.Format-List
. Creates lists of objects' properties.Format-Wide
. Depicts a single object's property spanning across the screen.Format-Table
. Presents the data in the form of a table.
For instance, to format the output into a table, run the following:
echo "Number", "Name", "Age", "Location" | Format-Table
The echo command is also useful in functions. For instance, write a function to test if a number is a prime number:
Function CheckIfNumberIsPrime($num) {
if ($num -lt 2) {
echo "$num is not a prime number"
}
else {
$isPrime = $true
for ($i = 2; $i -lt $num; $i++) {
if ($num % $i -eq 0) {
$isPrime = $false
break
}
}
if ($isPrime) {
echo "$num is a prime number"
}
else {
echo "$num is not a prime number"
}
}
}
To test the function, run:
CheckIfNumberIsPrime($num)
Replace ($num)
with a prime number, for example:
CheckIfNumberIsPrime(7)
The output confirms seven is a prime number. Next, try 135:
CheckIfNumberIsPrime(135)
The output verifies 135 is not a prime number.
Note: Check out our breakdown of differences between PowerShell and CMD.
Example of Write-Output in PowerShell
The Write-Output
cmdlet is a powerful command which prints text, objects, and formatted output to the console.
The basic Write-Output
syntax is:
Write-Output [object]
The object can be text, numbers, arrays, etc. The Write-Output
usage examples are the same as echo
.
For instance, run the following:
Write-Output "This is another sentence."
Using a function with Write-Output
does not differ from echo
. The function in the following example is the PrimeNumber?
function. It is the same as the earlier one, designed to identify prime numbers:
Function PrimeNumber?($num) {
if ($num -lt 2) {
Write-Output "$num is not a prime number"
}
else {
$isPrime = $true
for ($i = 2; $i -lt $num; $i++) {
if ($num % $i -eq 0) {
$isPrime = $false
break
}
}
if ($isPrime) {
Write-Output "$num is a prime number"
}
else {
Write-Output "$num is not a prime number"
}
}
Test the function with the number seven by running:
PrimeNumber?(7)
Next, test with 135:
PrimeNumber?(135)
The outputs confirm seven is a prime number while 135 is not.
Note: Learn how to use Command Prompt by utilizing our list of Windows CMD commands.
Write-Host vs. Echo
Write-Host
is a PowerShell cmdlet that prints text to the console for display purposes only. It does not return any value to the PowerShell engine. The primary purpose is to produce for-(host)-display-only output, such as printing colored text when prompting the user for input.
Write-Output
, on the other hand, writes data to the PowerShell pipeline. The output is displayed on the screen if there is no subsequent command in the pipeline to receive this data.
A key Write-Host
advantage is the customization capability. Write-Host
supports a broader range of formatting options.
To specify the output's foreground and background colors, use:
Write-Host "Text" -ForegroundColor [Color] -BackgroundColor [Color]
For instance, print a line This sentence is white with a magenta background:
Write-Host "This sentence is white with a magenta background" -ForegroundColor White -BackgroundColor Magenta
The output is displayed in the requested format.
Conclusion
After reading this article, you know how to use echo
, Write-Output
, and Write-Host
in PowerShell.
Next, learn how to set environment variables in Windows.