Bash eval
is a built-in shell command used to evaluate and execute strings as a shell command. eval
takes and treats arguments as a single command line for the shell to run as a Bash command.
The eval
command is useful when executing a command that contains a special operator or reserved keywords or in scripts with variables whose names are unknown until the script executes.
In this tutorial, you will learn to use the eval
command through hands-on examples.
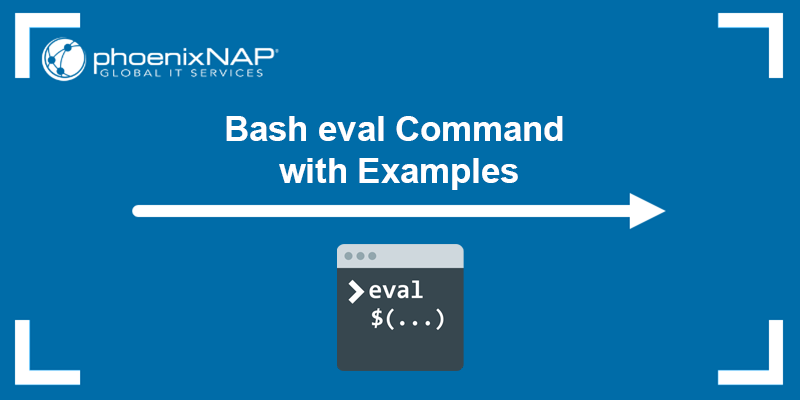
Prerequisites
- A system running Linux.
- Access to the terminal (Ctrl + Alt + T).
Bash eval Command Syntax
The eval
command has the following syntax:
eval [arguments]
Here, [arguments]
are the strings or variables that contain the command(s) you want to execute. The eval
command concatenates the arguments into a single string, parses the string as a shell command line, and executes it.
After executing the concatenated command line, eval
returns its exit status as the exit status of eval
. If there are no arguments or only empty arguments, eval
returns the exit status 0
.
Important: Be cautious when using eval
on a string you received from somewhere outside the script (from another user, through an API, etc.) which hasn't been properly validated or sanitized. If the string was not derived locally and programmatically, it might contain embedded malicious instructions and damage your system.
Bash eval Command Examples
The primary use of eval
is to interpret and execute dynamic or complex commands stored in strings or variables. This allows you to generate and run commands dynamically.
The following sections cover some hands-on eval
command examples.
Store Command in a Variable
Storing a command in a variable is useful, especially when you want to store it with an option or flag appended to the command. In the following example, we will store the ls command with the -l
option in a variable named var
:
var="ls -l"
eval "$var"
The eval
command evaluates the var
variable value as ls -l
and executes the command:
Command Substitution
In the following example, the eval
command substitutes the date command placed within a string stored in the command
variable. eval
evaluates the string and executes the result:
command="echo \$(date)"
eval "$command"
The output shows the date
command output, which was substituted using eval
.
Convert Input
Another example of eval
command use is to convert field values to lowercase. The following script uses eval
with the tr command to convert the values of the specified fields to lowercase characters.
Follow the steps below to convert:
1. Open the terminal and create a new Bash script. Use a text editor such as vi
/vim
:
vi convertinput.sh
2. Enter the following code:
#!/bin/bash
# Prompt the user to enter values
read -p "Enter value 1: " value1
read -p "Enter value 2: " value2
# Convert values to lowercase using eval
eval "value1=\$(tr '[:upper:]' '[:lower:]' <<< "$value1")"
eval "value2=\$(tr '[:upper:]' '[:lower:]' <<< "$value2")"
# Print the lowercase values
echo "Value 1 (lowercase): $value1"
echo "Value 2 (lowercase): $value2"
- The script prompts the user for input for each variable.
- The
tr '[:upper:]' '[:lower:]'
command within theeval
statements performs the conversion and replaces all uppercase characters with their lowercase equivalents. - After the conversion, the script prints the lowercase values for each field.
3. Save the script and exit vi:
:wq
4. Run the Bash script to test if it works:
bash convertinput.sh
Loops and Dynamic Commands
The eval
command can generate and execute commands inside a loop dynamically. In the following example, eval
evaluates the "echo 'Loop iteration $i'"
string for each iteration and dynamically substitutes the value of $i
.
Follow the steps below:
1. Create a new script and paste the following code:
#!/bin/bash
for i in {1..3}; do
eval "echo 'Loop iteration $i'"
done
2. Save the script and run it:
Each line in the output corresponds to one iteration of the loop, with the value of i
substituted into the output.
Analyze Field Values
Use the eval
command to evaluate and analyze field values dynamically. In the following example, we use a script that prompts the user to enter values and then analyzes them:
1. Create a script and paste the following code:
#!/bin/bash
# Prompt the user to enter field values
read -p "Enter field 1: " field1
read -p "Enter field 2: " field2
read -p "Enter field 3: " field3
# Analyze field values using if statements
if [ "$field1" = "Value1" ]; then
eval "echo 'Field 1 is Value1'"
elif [ "$field1" = "Value2" ]; then
eval "echo 'Field 1 is Value2'"
else
eval "echo 'Field 1 is neither Value1 nor Value2'"
fi
if [ "$field2" -gt 10 ]; then
eval "echo 'Field 2 is greater than 10'"
else
eval "echo 'Field 2 is less than or equal to 10'"
fi
if [[ "$field3" =~ ^[A-Za-z]+$ ]]; then
eval "echo 'Field 3 contains only letters'"
else
eval "echo 'Field 3 contains characters other than letters'"
fi
2. Save and run the script.
After a user enters three field values (field1
, field2
, field3
), the script uses the eval
command within if statements to analyze each field and print the corresponding analysis message:
The eval
command evaluates the echo command statements dynamically based on the user input and executes them accordingly.
Access Variable Within Variable
Use the eval
command to assign a value to a variable and then assign that variable's name to another variable. Through eval
, you can access the value held in the first variable by using the name stored in the second variable.
Follow these steps:
1. Create a new script and paste the following code:
#!/bin/bash
title="Welcome"
name=title
command="echo"
eval $command \${$name}
2. Run the script to test if it works:
Running the script outputs the text from the title
variable even though the eval
command is using the name
variable. eval
looks at the value held inside the variable whose name is stored in the name
variable.
Store SSH Configuration in Shell
Use eval
to evaluate the ssh-agent
program output and store SSH keys and passwords in the shell. Normally, when you run ssh-agent
, it executes in a subshell and cannot affect the parent shell environment.
Note: Read our tutorial and see how to use public key authentication with SSH.
Consequently, the configuration is unknown to the current terminal session or any new session you create. It means that if you exit the shell or try to initiate a new SSH connection, you will always be prompted for your SSH key and password.
However, when you use eval
, the command adds the configuration to the current shell's environment. Run the following to test:
eval $(ssh-agent)
The command evaluates the ssh-agent
output and automatically uses the credentials each time to create a new connection until you log out of the operating system or reboot the machine.
Note: Learn how to concatenate strings in Bash to build longer strings by appending one string to the end of another.
Conclusion
The eval
command in Bash is a powerful tool that allows you to evaluate and execute strings as shell commands dynamically. It is helpful when dealing with complex or dynamically generated commands stored in variables or strings.
Next, learn how to increment or decrement a variable in Bash, how to compare strings in Bash, or check out our ultimate Bash commands guide that comes with a free PDF cheat sheet.