Bash is one of the most versatile command-line scripting languages. Bash arrays are a well-known and familiar data structure. However, the lesser-known associative arrays provide even more flexibility for handling data as key-value pairs.
Learning to use associative arrays will improve your Bash scripting skills, especially in complex data organization and parsing.
In this article, you will learn about associative arrays in Bash and their practical application.
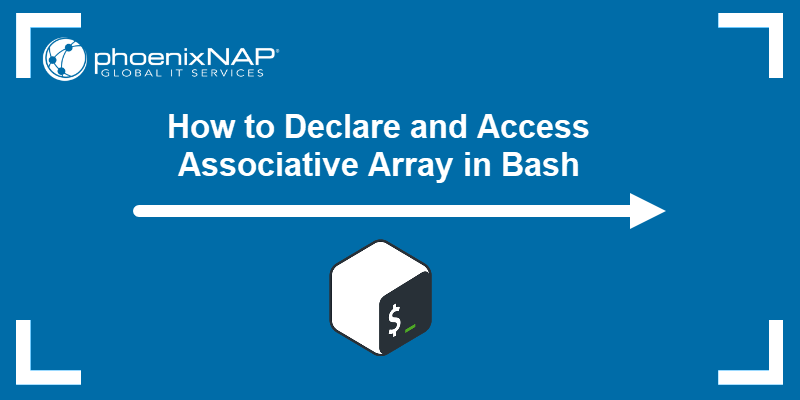
Prerequisites
- Access to the command line/terminal.
- A text editor to write Bash scripts for testing.
- Bash version 4 or higher.
What is Associative Array in Bash?
An associative array in Bash is a data structure for storing key-value pairs. Every key is unique and has an associated value. Associative arrays provide a way to index and retrieve values based on corresponding keys.
Associative arrays first appeared in Bash version 4. Check the Bash version on your system using the following environment variable:
echo $BASH_VERSION
The BASH_VERSION
variable stores the currently running Bash shell version.
If the command does not print any output, Bash is either not running on the system or the variable has changed. Try pressing CTRL+X
CTRL+V
as another method to show the current Bash version.
How to Use Associative Arrays in Bash
There are several elementary procedures to know when working with Bash associative arrays. These include the following:
- Declaring an array.
- Assigning values.
- Accessing values.
- Iterating over an array's elements.
The sections below cover these procedures through hands-on examples.
Declare and Add Elements to Associative Array
Use the Bash declare keyword to create an empty associative array in Bash. For example:
declare -A example_array
The -A
tag tells the declare
command to create an associative array if supported. The declaration does not print an output.
To populate the array, enter new values one by one:
example_array["key1"]="value1"
example_array["key2"]="value2"
example_array["key3"]="value3"
Alternatively, populate the array immediately during initialization:
declare -A example_array=(["key1"]="value1", ["key2"]="value2", ["key3"]="value3")
The second method is harder to read but creates an array and elements in a single line. In both cases, the commands do not print a response.
Create Read-Only Associative Array
To create a read-only associative array, use the following command:
declare -rA example_array
After initializing the array, modifying or adding elements is impossible. Use read-only associative arrays to store unchanging key-value pairs.
Print Keys and Values
To print the values of an associative array, use the echo or printf command and reference all the array elements. For example:
echo ${example_array[@]}
The at symbol (@
) accesses all array elements and prints only the values. To print the associative array keys, use the following:
echo ${!example_array[@]}
The exclamation mark (!
) retrieves array indices, which are the array keys in this case.
The keys and values do not print in the order of declaration.
Find Length of Associative Array
To check the array length, prefix the array with the hash symbol (#) and print all the array elements. For example:
echo ${#example_array[@]}
The command prints the element count to the console.
Access Values
To access a specific value for a key, use the following syntax:
echo ${example_array["key1"]}
The command prints the corresponding value for the provided key.
Append New Values
To add new elements to an existing associative array, provide a key-value pair and add it to the array:
example_array["new_key"]="new_value"
Alternatively, append using the following syntax:
example_array+=(["new_key"]="new_value")
If the key is already in the array, the operation overwrites the existing value with the provided new one.
Iterate Over an Array
Use a Bash for loop script or run a for loop in the terminal to iterate over the array keys or values. For example, to print corresponding key-value pairs, use a for
loop and iterate over the keys. Here is what that example would look like in the terminal:
for key in ${!example_array[@]}
do
echo "${key}, ${example_array[${key}]}"
done
Check if a Key Exists
A simple way to check if an element exists in an array is to use a conditional if
statement. For example:
if [[ -n "${example_array["key1"]}" ]]
then
echo "True"
else
echo "False"
fi
The -n
tag checks if the array returns a non-zero element when searching for the provided key in the associative array.
Clear Associative Array
To clear an array from a key-value pair, use the unset
command and provide the appropriate key. For example:
unset example_array["key1"]
Alternatively, to clear the associative array from all values, redeclare it using the same name:
declare -A example_array
Creating a new variable with the same name overwrites the existing one.
Delete Associative Array
To delete an associative array, use the unset
command and provide the array name:
unset example_array
The command removes the array variable and all the elements.
Conclusion
After reading this guide, you learned about associative arrays in Bash and how to use them. The feature appears in Bash version 4+ and aims to provide a key-value pair data type.
Keep in mind that the syntax for associative arrays differs between different Linux shells.