Syntax highlighting is a feature in text editors and integrated development environments (IDEs) that visually distinguishes code elements using color and formatting. By applying different styles to keywords, variables, strings, and other components, it enhances code readability and helps developers quickly identify syntax errors or patterns.
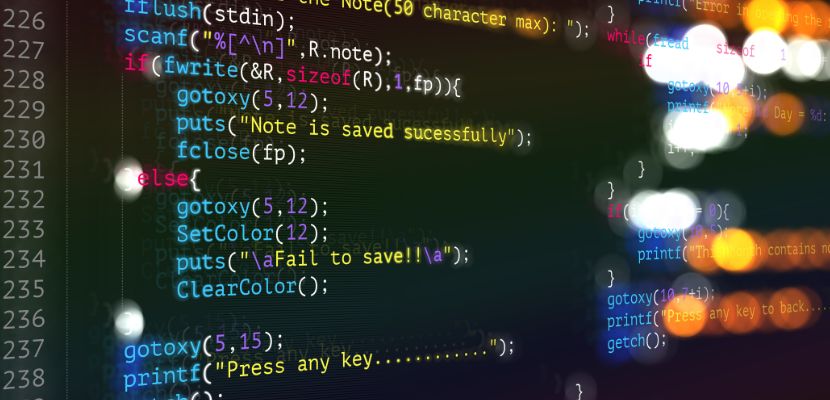
What Is Syntax Highlighting?
Syntax highlighting is a functionality in text editors and integrated development environments (IDEs) that applies color coding and visual differentiation to various elements of programming and markup languages. It identifies and highlights specific language components, such as keywords, variables, operators, strings, comments, and data types, based on predefined syntax rules.
This visual differentiation enhances code readability, making it easier for developers to understand the structure and logic of the code at a glance. Additionally, syntax highlighting helps in error detection by visually flagging incorrect or incomplete code, allowing developers to identify issues more efficiently during the coding process. By improving both clarity and debugging efficiency, syntax highlighting serves as a critical tool for writing, maintaining, and understanding code in software development.
The Elements of Syntax Highlighting
Syntax highlighting involves several key elements that work together to enhance code readability and assist in identifying patterns or errors. These elements are tailored to the specific programming or markup language being used, ensuring accurate and relevant visual feedback. Below are the primary components of syntax highlighting and their roles:
- Keywords. Keywords are reserved words in a programming language that have predefined meanings, such as if, else, while, or function. Syntax highlighting distinguishes these words with unique colors or styles, helping developers quickly identify control flow and language-specific constructs.
- Variables. Variables represent storage locations for data. Highlighting variables in a distinct style makes it easier to differentiate between identifiers and reserved words, allowing developers to track data flow and usage throughout the code.
- Strings. Strings, typically enclosed in quotes, represent text values. They are often highlighted in a specific color to stand out from the surrounding code, helping to prevent errors such as mismatched quotes or incorrect concatenations.
- Operators. Operators, including mathematical (+, -, *, /), logical (&&, ||), and comparison operators (==, !=), are essential for defining expressions and conditions. Highlighting them ensures they are easily distinguishable within complex expressions.
- Comments. Comments are annotations in code that are not executed but provide context or explanation for the logic. By using a unique style, often a muted color, syntax highlighting visually separates comments from executable code, reducing distractions while maintaining clarity.
- Data types. Data types such as int, float, string, or Boolean define the kind of data a variable can hold. Highlighting these terms helps reinforce their importance in variable declarations and function definitions.
- Functions and methods. Functions and methods represent reusable blocks of code. Highlighting their names when they are defined or called helps in distinguishing between custom code and built-in language features.
- Errors and warnings. Syntax errors, such as missing brackets or incorrect declarations, are often highlighted in contrasting colors or underlined to draw attention to potential issues. This immediate feedback aids in efficient debugging.
Many text editors and IDEs allow users to customize syntax highlighting themes, tailoring the colors and styles to their preferences for improved comfort and productivity. Furthermore, modern syntax highlighting tools support multiple programming languages, automatically adjusting the color schemes and rules based on the language being used.
Example of Syntax Highlighting
Here's an example of syntax highlighting using Python code. The color scheme is typical of many IDEs and text editors:
# Import necessary library
import math # Keyword (highlighted in blue or bold)
# Define a function
def calculate_area(radius): # 'def' is a keyword, function name is often bold
if radius <= 0: # 'if' is a keyword, operators like '<=' are highlighted
return "Invalid radius" # String in green or orange
area = math.pi * radius**2 # Variables in white, constants in blue
return area # 'return' keyword highlighted in blue
# Call the function
result = calculate_area(5) # Function calls in bold or italic
print(f"The area is: {result}") # f-strings in green, variables inside curly braces in white
Breakdown of Highlighting:
- Keywords: import, def, if, return, print (often blue or bold).
- Strings: "Invalid radius", f"The area is: {result}" (green or orange).
- Functions: calculate_area, print (italicized or bold).
- Variables: radius, area, result (standard white or uncolored).
- Operators: <=, *, ** (highlighted in distinct colors like red or yellow).
Features of Syntax Highlighting
Syntax highlighting enhances the coding experience by offering a range of features designed to improve readability, productivity, and error detection. Below are the key features, along with explanations:
- Color coding. Syntax highlighting uses distinct colors to differentiate between code elements, such as keywords, variables, strings, comments, and operators. This visual separation makes it easier to identify and understand the structure of the code.
- Language-specific rules. Highlighting is tailored to the syntax of specific programming languages. This ensures accurate recognition and styling of language-specific keywords, constructs, and conventions, making it adaptable to various coding environments.
- Customizable themes. Many editors allow users to customize color schemes and styles to suit their preferences. This feature accommodates accessibility needs, such as color blindness, and personal aesthetic choices.
- Dynamic updating. Syntax highlighting updates in real time as the user types, ensuring that new code or changes are immediately styled according to the defined syntax rules. This feature is particularly helpful for detecting errors while coding.
- Error highlighting. Some syntax highlighters visually indicate syntax errors, such as mismatched parentheses, missing semicolons, or invalid keywords, using underlines or distinct colors. This immediate feedback aids in debugging and correcting mistakes.
- Support for multiple languages. Advanced syntax highlighters can recognize and apply styles to different programming languages within the same file, such as HTML, CSS, and JavaScript in a web development project. This feature is useful for working with complex, multilanguage codebases.
- Integration with code editors and IDEs. Syntax highlighting is integrated into most modern text editors and IDEs, offering seamless support for a wide range of development tools and platforms.
- Token recognition. Highlighters identify and style tokens, such as functions, classes, methods, and variables, based on their role and placement within the code, enhancing comprehension of the codeโs logic and flow.
- Comment differentiation. Comments are highlighted in muted colors or italics to distinguish them from executable code. This ensures clarity while reading the code without distracting from its main logic.
- Bracket matching. Some syntax highlighters assist with pairing brackets or parentheses by highlighting matching pairs, reducing errors in nested or complex expressions.
- Performance optimization. Modern syntax highlighters are optimized for large code files, using efficient algorithms to ensure smooth scrolling and editing without lag.
What Is the Benefit of Syntax Highlighting?
The primary benefit of syntax highlighting is improved code readability and developer productivity. By using colors and visual styles to differentiate elements such as keywords, variables, and operators, syntax highlighting helps developers quickly understand the structure and logic of their code. This reduces cognitive load, making it easier to navigate complex codebases.
Additionally, syntax highlighting assists in error detection, as visual cues can help identify issues like missing syntax elements, mismatched brackets, or incorrect keywords. This immediate feedback minimizes debugging time and allows developers to focus on problem-solving rather than searching for mistakes.
Uses of Syntax Highlighting
Syntax highlighting serves a variety of purposes in software development, making it an essential tool for developers. Below are the key uses and explanations of how it enhances programming workflows:
- Improving code readability. Syntax highlighting applies colors and formatting to different code elements, making the structure of the code visually clear. Developers can easily distinguish between functions, keywords, variables, and comments, which is particularly useful when navigating through complex or lengthy codebases.
- Facilitating debugging. Highlighting provides immediate visual feedback on syntax errors, such as missing brackets, invalid keywords, or improperly terminated strings. This helps developers quickly spot and fix mistakes, reducing the time spent on debugging.
- Enhancing learning and understanding. For beginners, syntax highlighting can make learning new programming languages easier by visually categorizing elements like keywords and functions. Visual differentiation helps learners quickly understand language syntax and structure.
- Increasing productivity. By reducing the effort needed to read and comprehend code, syntax highlighting allows developers to focus on problem-solving and implementing features, boosting overall development productivity.
- Supporting multilingual projects. Syntax highlighting is adaptable to various programming languages, enabling developers to work seamlessly with different technologies in the same environment. This is particularly beneficial in projects involving multiple languages, such as combining HTML, CSS, and JavaScript.
- Improving collaboration. When working in teams, syntax highlighting ensures that code remains clear and readable for all team members, even if they are unfamiliar with certain parts of the project. This shared understanding improves collaboration and reduces miscommunication.
- Encouraging best practices. Highlighting can visually emphasize proper coding practices, such as consistent variable naming and correct use of keywords. It serves as a subtle guide for adhering to language conventions and maintaining code quality.
- Assisting with code reviews. During code reviews, syntax highlighting helps reviewers quickly identify critical sections of code, such as changes to logic, function definitions, or control structures, making the review process more efficient.
How to Create a Syntax Highlighter?
Creating a syntax highlighter involves defining a set of rules for recognizing patterns in text and applying corresponding styles to those patterns. The process typically includes parsing the text, identifying language-specific elements, and rendering them with appropriate formatting. Here's a detailed explanation of how to create a syntax highlighter:
1. Understand the Basics
A syntax highlighter works by recognizing specific patterns in the text, such as keywords, variables, strings, or comments, and then applying visual styles (like colors or font styles) to these patterns.
2. Choose the Environment
Decide where the syntax highlighter will be used:
- Text editors/IDEs. Examples include Visual Studio Code or Sublime Text.
- Web applications. Highlighting code in a browser, often for documentation or live editors.
- Standalone programs. Custom-built tools or CLI utilities.
3. Select or Implement a Text Parsing Engine
To detect syntax elements, use one of these methods:
- Regular expressions. Define regex patterns for language elements (e.g., keywords, strings, comments).
- Tokenization. Split the code into tokens and classify each based on predefined rules.
- Abstract syntax tree (AST). For more advanced parsing, use a language parser to generate an AST and map syntax elements.
4. Define Syntax Rules
Create rules for each language element you want to highlight. For example:
- Keywords: if, else, function, return
- Operators: +, -, *, /, =
- Strings: Enclosed in " " or ' '
- Comments: Single-line (// or #) and multi-line (/* */)
Example regex for strings:
"([^"\\]*(\\.[^"\\]*)*)"
5. Apply Styles
Determine how to render each recognized element. Styles can include:
- Text color (e.g., blue for keywords, green for strings)
- Font weight (e.g., bold for function names)
- Background color or underlines (for errors or warnings)
In a web-based highlighter, you can use CSS classes:
<span class="keyword">if</span>
.keyword {
color: blue;
font-weight: bold;
}
6. Integrate the Highlighter
- For text editors. Create a plugin or extension that hooks into the editor's API to apply your syntax rules.
- For web applications. Use a library like Prism.js or Highlight.js, or create a custom solution using JavaScript to parse and style the code dynamically.
- For CLI tools. Use libraries like Pygments (Python) or custom parsing logic to colorize terminal output.
7. Optimize for Performance
Large code files or real-time typing require efficient parsing. Implement optimizations such as:
- Incremental parsing. Update only the modified parts of the text.
- Caching. Reuse results for unchanged sections.
8. Test with Multiple Languages
Ensure your syntax highlighter works accurately across different languages by testing with diverse code samples. Add support for multiple languages by defining separate sets of rules for each.
What Is the Disadvantage of Syntax Highlighting?
While syntax highlighting offers numerous advantages, it also has some potential disadvantages:
- False sense of understanding. Syntax highlighting can make code appear easier to understand than it actually is, especially for beginners. The visual cues might lead to superficial comprehension without a deeper understanding of the underlying logic or structure.
- Overreliance on visual cues. Developers might become overly dependent on the colors and formatting provided by syntax highlighting. When these visual aids are absent, such as in plain text environments, reading and debugging code becomes more challenging.
- Inconsistent styles across tools. Different editors and IDEs may use varying color schemes or syntax rules, leading to inconsistencies. Switching between tools might cause confusion or require time to adapt to a new highlighting style.
- Performance overhead. In large codebases or real-time environments, syntax highlighting can slow down the editor, especially if the implementation is not optimized. This can lead to lag or decreased responsiveness during coding.
- Limited help with logic errors. Syntax highlighting only identifies syntax-related issues and provides visual organization. It does not assist with detecting logical or semantic errors, which are often more critical and harder to debug.
- Cluttered appearance. Excessive use of colors or overly detailed highlighting can make code look cluttered and harder to read. Poorly chosen color schemes or too many distinct styles can overwhelm the developer instead of aiding clarity.
- Accessibility issues. Developers with color blindness or other visual impairments may find certain color schemes difficult to interpret. If the syntax highlighter does not support customization, it might create barriers for such users.
How Do I Remove Syntax Highlighting?
Removing syntax highlighting depends on the context in which youโre working. Below are some common scenarios and methods to disable or remove syntax highlighting:
1. In a Text Editor or IDE
Most modern text editors and IDEs allow you to disable syntax highlighting through settings or preferences.
- Steps:
- Open the editor's settings/preferences menu.
- Look for an option related to syntax highlighting or color themes.
- Select a "Plain Text" theme or disable syntax highlighting altogether.
- Examples:
- In Visual Studio Code:
- Set the language mode to "Plain Text" via the bottom-right status bar.
- In Sublime Text:
- Go to View > Syntax and choose Plain Text.
2. In a Web-Based Highlighter (e.g., Markdown or HTML Viewer)
Web applications or documentation tools often use libraries like Prism.js or Highlight.js for syntax highlighting.
Steps:
- Remove the library's script or CSS reference from the HTML file.
- If dynamically applied, ensure the code snippet is not processed by the highlighter by modifying the related class or ID.
3. Using Command-Line Tools
If youโre viewing syntax-highlighted output in a terminal, you can:
- Pipe the output to cat or another tool that strips formatting:
command | cat
- Disable the syntax highlighting option in your command-line tool:
- For ls, use the --color=never flag:
ls --color=never
4. In Markdown Files or Code Blocks
If syntax highlighting is applied to fenced code blocks in Markdown:
- Remove the language specifier after the opening backticks:
```python
# Highlighted
Change to:
```markdown
No highlighting
5. In Custom Scripts or Applications
If youโve implemented syntax highlighting in your application using libraries:
- Disable the feature in the configuration settings.
- Remove the functions or classes responsible for applying highlighting.
6. Using Word Processors or Plain Text Viewers
If code with syntax highlighting is copied into a rich-text editor, the formatting (colors, styles) might be retained. To remove it:
- Paste the code into a plain text editor (e.g., Notepad).
- Copy and paste it back into your target document.
What Is the Difference Between Semantic and Syntax Highlighting?
Syntax highlighting focuses on visually distinguishing code elements based on their syntactic role in the programming language, such as keywords, strings, operators, and comments, regardless of their meaning or usage in the context of the code.
Semantic highlighting, on the other hand, provides deeper context-aware coloring by considering the meaning and behavior of elements within the code, such as differentiating between variables based on their scope, type, or role (e.g., local vs. global variables, function vs. method calls).
While syntax highlighting enhances readability at a surface level, semantic highlighting offers a more precise and intelligent visual distinction, aiding in understanding the codeโs functionality and structure.