First fit allocation is a memory management technique in which the system allocates the first available block of memory that is large enough to satisfy the requested size.
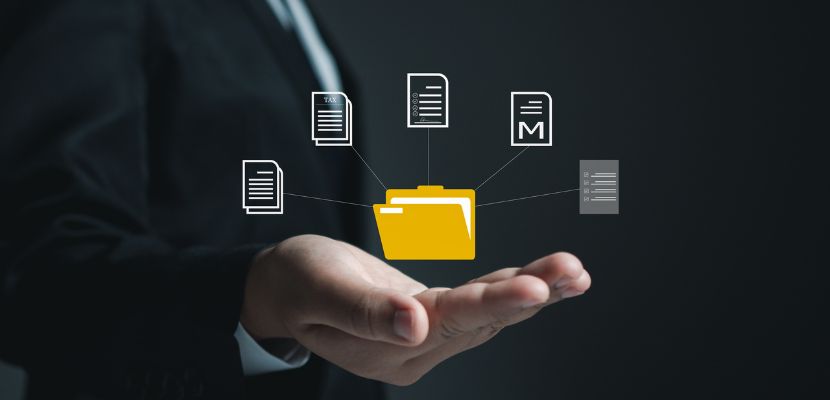
What Is First Fit Allocation?
First fit allocation is a memory management strategy used by operating systems to assign memory blocks to processes. In this approach, when a process requests memory, the system searches through the available memory blocks and allocates the first block that is large enough to fulfill the request. The search for a suitable memory block starts from the beginning of the list of free memory areas and continues sequentially until a block that meets the size requirements is found. Once this block is allocated, the system proceeds with its operation, and the allocated memory is marked as unavailable for other processes.
While first fit allocation is relatively fast because it stops searching once a suitable block is located, it has some limitations. Over time, this method can lead to fragmentation, as smaller gaps of unused memory might accumulate between allocated blocks. These gaps may not be large enough to accommodate future memory requests, even though there is enough total unused memory in the system. This reduces overall memory efficiency, but the simplicity and speed of the method often make it a practical choice in environments where speed is prioritized over memory optimization.
What Is a First Fit Allocation Example?
Hereโs an example of how first fit allocation works:
Imagine a system with the following free memory blocks of various sizes:
- Block 1: 100 KB
- Block 2: 250 KB
- Block 3: 50 KB
- Block 4: 200 KB
- Block 5: 300 KB
Now, suppose a process requests 150 KB of memory.
Step-by-Step Process of First Fit Allocation:
- The system will first check Block 1 (100 KB), but it is too small to accommodate the request, so it moves on to the next block.
- Next, it checks Block 2 (250 KB). Since this block is large enough to satisfy the 150 KB request, it allocates this block to the process.
- The process is now allocated 150 KB from Block 2, and Block 2โs remaining space (100 KB) is still free and available for future use.
In this example, the system did not check Block 3, Block 4, or Block 5 because it found the first block that was large enough (Block 2). This is the essence of first fit allocation: it allocates memory from the first available block that meets the required size without further consideration for the remaining free space in other blocks or whether those blocks could fit the request.
First Fit Allocation Uses
First fit allocation is commonly used in scenarios where speed and simplicity are prioritized over the efficient use of memory. Here are some common uses:
- Operating systems for process memory management. In many operating systems, first fit allocation is used to allocate memory for running processes. Since it is relatively fast, it helps in efficiently managing memory allocation requests without significantly impacting system performance. This is especially useful in real-time or embedded systems where allocation speed is crucial.
- Embedded systems. Embedded systems often have limited resources and require fast memory allocation techniques. First fit allocation, due to its simplicity and speed, is used to manage memory in such environments.
- Virtual memory management. In systems where virtual memory is used, first fit allocation can be applied to allocate physical memory to processes. While it may lead to fragmentation, it is often used in conjunction with other techniques (such as paging or segmentation) to manage memory efficiently.
- Memory allocation in simple applications. For applications where memory requirements are predictable and the system is not heavily stressed, first fit allocation can be used. These applications do not need complex memory management and can tolerate some degree of fragmentation that comes with this method.
- Dynamic memory allocation in low-level programming. In low-level programming, such as with C or C++, first fit allocation is often used in dynamic memory management (via malloc or free functions). It helps allocate memory blocks from a pool and is straightforward for managing small to medium-scale memory requests in a simple heap structure.
How to Optimize First Fit Allocation?
Optimizing first fit allocation involves the reduction of fragmentation and improvement of memory utilization without significantly sacrificing its simplicity or speed. Here are some strategies that can help optimize first fit allocation:
- Coalescing free memory blocks. One of the most common issues with First fit allocation is fragmentation, where small unused gaps accumulate between allocated blocks. To optimize this, coalescing can be applied. When a block of memory is freed, the system should check neighboring free blocks and combine them into a larger contiguous free block. This helps reduce fragmentation and increases the chances of finding larger free blocks for future allocations.
- Maintaining a sorted list of free blocks. Sorting the free blocks by size can improve the efficiency of memory allocation. When the free blocks are sorted in increasing order, the system can more quickly locate a suitable block, as the first block found will be the smallest that fits the request. This reduces the chances of wasting large memory areas with smaller allocation requests.
- Using a binning system. Implementing a binning or bucketing system where free memory blocks are grouped by size ranges further optimizes first fit allocation. When allocating memory, the system first checks the bin corresponding to the requested size and then searches within it. This reduces the need to scan through all available blocks, making the allocation process faster and more efficient.
- Splitting blocks for better utilization. If a free block is larger than necessary, first fit allocation can split the block into two: one for the current allocation and the other as a smaller free block for future use. This helps in making better use of memory, as the leftover space is not wasted, and it helps to avoid large gaps of unused memory.
- Using memory pools. Memory pools are pre-allocated regions of memory that are divided into fixed-size blocks. By using memory pools for allocating memory of specific sizes, the need to search through the free memory list can be minimized, and fragmentation can be controlled. This method is especially useful when memory requirements are predictable, and the system frequently allocates similar-sized blocks.
- Periodic compaction. Over time, memory fragmentation can become severe, especially with first fit allocation. Implementing periodic memory compaction, where the system periodically moves allocated memory blocks to consolidate free space, can help optimize memory utilization. This reduces fragmentation but at the cost of some overhead, so it needs to be done carefully to balance performance.
- Allocating larger memory blocks at the start. When the system initially allocates memory, it can prioritize larger blocks for larger memory requests. This approach helps reduce fragmentation because larger blocks are less likely to be split into too many small gaps, making more room for subsequent allocations.
The Advantages and the Disadvantages of First Fit Allocation
The First fit allocation method offers a simple and fast approach to memory management, making it a popular choice for many systems. However, like any technique, it comes with its own set of advantages and disadvantages.
What Are the Advantages of First Fit Allocation?
The advantages of First fit allocation include:
- Simplicity and speed. First fit is straightforward to implement, and it quickly allocates memory by searching for the first suitable block.
- Low overhead. Since the algorithm stops as soon as it finds a suitable block, it minimizes the computational overhead compared to other strategies like best fit or worst fit, which might require searching through all available memory blocks.
- Effective for small to medium-sized systems. In systems with predictable and modest memory requirements, first fit allocation works efficiently without the need for complex memory management mechanisms.
- Less complex memory management. First fit doesn't require maintaining complex data structures or performing complicated calculations, reducing the complexity of memory management systems.
- Good for real-time systems. In real-time applications where memory allocation speed is critical, first fit provides a quick solution with minimal delays, as it allocates memory as soon as a suitable block is found.
What Are the Disadvantages of First Fit Allocation?
While first fit allocation offers simplicity and speed, it comes with several disadvantages:
- Fragmentation. Over time, first fit allocation can lead to both external and internal fragmentation. External fragmentation occurs when there are many small, unused gaps between allocated memory blocks, while internal fragmentation happens when allocated blocks are larger than necessary. These fragmented spaces reduce the overall efficiency of memory usage.
- Inefficient memory usage. Since first fit simply selects the first block that is large enough to satisfy the request, it may leave smaller gaps in memory that could have been better utilized with a different allocation strategy. This can lead to wasted space, especially in systems with many varying allocation sizes.
- Increased search time. Although first fit can be fast, as the system continues to allocate and free memory blocks, the list of free blocks may become more disordered. In cases where there are many small, scattered free blocks, the time it takes to find the first suitable block increases, affecting overall system performance.
- Poor handling of large allocations. First fit allocation tends to prioritize quick allocation over optimal use of memory. As a result, it might not be efficient when handling large memory requests, as it might end up allocating smaller, fragmented blocks that are not ideal for the requested size.
- Lack of optimization. First fit does not consider the best available block for allocation, meaning it doesn't attempt to minimize fragmentation or optimize the usage of memory. It simply takes the first block that fits, which may not always lead to the most efficient memory management in the long term.
First Fit vs. Best Fit vs. Worst Fit Allocation: What Are the Differences?
Hereโs a comparison of first fit, best fit, and worst fit allocation in a table format:
Criteria | First fit | Best fit | Worst fit |
Allocation strategy | Allocates the first available block that fits the memory request. | Allocates the smallest block that is large enough to fit the request. | Allocates the largest available block, aiming to leave the largest possible leftover space. |
Speed | Fastest, as it stops searching after finding the first fit. | Slower than first fit, as it requires checking all available blocks to find the best fit. | Slower than first fit, as it also requires searching for the largest block. |
Fragmentation | Can lead to external fragmentation due to scattered small gaps. | Reduces external fragmentation more effectively than first fit but may still cause it. | May lead to internal fragmentation, as the leftover space is often very large. |
Efficiency | Less efficient in terms of memory usage due to potential wasted space in scattered blocks. | More efficient than first fit, as it aims to minimize wasted space. | Can lead to inefficient memory usage, as large gaps are left unused in large blocks. |
Memory utilization | Memory utilization can degrade over time as smaller gaps accumulate. | Memory utilization is better as it reduces smaller gaps, but can still lead to fragmentation. | Poor memory utilization, especially when large gaps are left unused. |
Best use case | Best suited for environments where allocation speed is prioritized over memory efficiency. | Suitable for systems where memory efficiency is more important than allocation speed. | Often used when trying to avoid fragmentation in small allocations, but not ideal for large systems. |
Handling of large allocations | Large allocations may end up in smaller blocks, leading to fragmentation. | Large allocations are handled better as the smallest fit is sought, but can still result in fragmentation. | Large allocations might cause large leftover spaces, resulting in inefficient use of memory. |