Declarative code is a programming paradigm that focuses on describing what the program should accomplish rather than how it should be done.
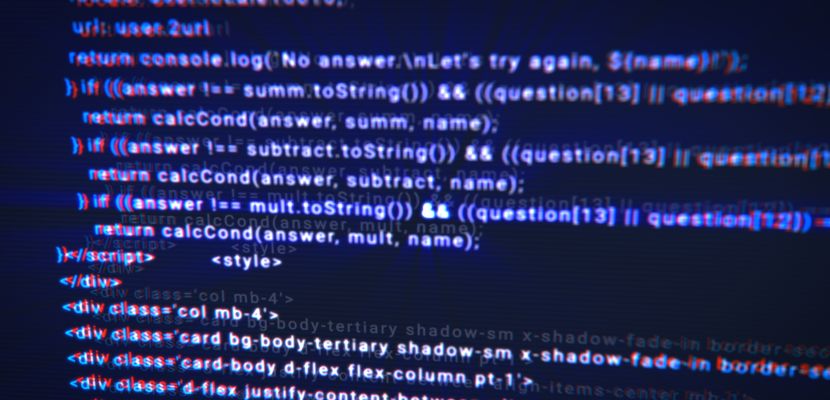
What Is Meant by Declarative Code?
Declarative code is a style of programming where the developer expresses the logic of computation without explicitly specifying the control flow or the steps required to achieve the desired result. Unlike imperative programming, which focuses on describing the sequence of operations needed to achieve a goal, declarative programming abstracts the process, allowing the developer to define what the outcome should be rather than how to achieve it.
This means that in declarative programming, the emphasis is on describing the properties or state of the system at various points in time, rather than providing a step-by-step procedure for how to modify that state. Declarative code often leads to simpler, more readable programs because it abstracts away the complexities of control structures, allowing the programmer to focus on the desired end results.
Declarative Code Examples
Here are a few examples of declarative code in different contexts:
- SQL (Structured Query Language)
SELECT name, age FROM users WHERE age > 30;
In this example, the code specifies what data to retrieve (name and age from users) and under what condition (age greater than 30), without detailing how the database should search, filter, or retrieve the data.
- HTML (HyperText Markup Language)
<h1>Welcome to My Website</h1>
<p>This is a sample page.</p>
Here, the developer describes the structure of the webpage, such as the presence of a heading and a paragraph. There's no need to specify how the browser should render the elements; it is abstracted away.
- CSS (Cascading Style Sheets)
h1 {
color: blue;
}
p {
font-size: 16px;
}
In this CSS example, the code declares the style for the h1 and p elements (blue color and font size, respectively), without describing the low-level process of how the browser should apply the styles.
- Functional Programming (Haskell)
sumList :: [Int] -> Int
sumList xs = sum xs
In Haskell, the code focuses on the whatโsumming the list of integersโwithout specifying the exact iteration process. The sum function takes care of the details of how the summing is done.
- React JSX (JavaScript Library for UI)
<button onClick={handleClick}>Click me</button>
This declarative code describes the UI element (a button) and the event handling logic (onClick). The developer specifies the desired outcome, which is a button with an event handler, but not the exact implementation of how the event is managed. React will handle the details.
Declarative Code Uses
Declarative code is used in a variety of domains and scenarios, offering advantages like simplicity, expressiveness, and abstraction from implementation details. Here are some key uses of declarative code:
- Database queries (SQL). SQL is one of the most common examples of declarative programming. In SQL, you specify what data you want (e.g., columns, tables, conditions) without needing to specify how the database should retrieve that data. The underlying database engine optimizes the execution, which allows developers to focus on the logic rather than the implementation details.
Example:
SELECT * FROM customers WHERE age > 30;
This query defines the data requirementsโretrieving all columns for customers whose age is greater than 30โwithout specifying how the database engine should perform the search.
- Web page layout (HTML/CSS). HTML and CSS are declarative languages used to structure and style web pages. HTML defines the structure of the page (such as headings, paragraphs, images) while CSS specifies how those elements should be styled (like colors, sizes, and positions). Developers declare the desired layout or appearance without instructing the browser how to render these elements.
Example:
<div class="header">Welcome</div>
In CSS:
.header {
font-size: 24px;
color: blue;
}
- Functional programming. Functional programming languages like Haskell, Scala, and parts of JavaScript focus on declarative programming. In these languages, functions are often written as expressions that describe transformations on data, rather than as sequences of steps. This leads to more concise, readable, and maintainable code that emphasizes immutability and stateless computation.
Example in Haskell:
map (*2) [1,2,3,4] -- [2,4,6,8]
This declares the desired transformation (multiplying each element by 2) rather than specifying how the iteration over the list should happen.
- UI frameworks (React, Vue.js). In UI frameworks like React or Vue.js, developers declare the structure of the user interface in terms of components, properties, and states. The framework takes care of the rendering and updates the UI as necessary. The declarative approach helps to avoid dealing with low-level DOM manipulations and event-handling code explicitly.
Example in React:
<button onClick={handleClick}>Click me</button>
Here, you declare that a button should appear, and React takes care of handling the event and rendering the UI updates when the button is clicked.
- Infrastructure as Code (IaC). Tools like Terraform, Ansible, and CloudFormation use declarative code to define infrastructure. In IaC, developers describe the desired infrastructure state, and the tool ensures the infrastructure matches that state. For example, you might declare that a virtual machine with certain properties should exist, and the tool will manage the process of creating or updating it.
Example in Terraform:
resource "aws_instance" "example" {
ami = "ami-123456"
instance_type = "t2.micro"
}
This defines the desired state for the virtual machine, and Terraform handles creating or modifying the instance.
- Data transformation (XSLT, LINQ). In data transformation scenarios, languages like XSLT or LINQ (Language Integrated Query in C#) allow declarative transformations of data from one format to another, like from XML to HTML or querying collections in memory. Instead of describing how to iterate over the data and perform the transformation, developers declare what the transformation should produce.
Example in XSLT:
<xsl:template match="book">
<h2><xsl:value-of select="title" /></h2>
</xsl:template>
- Declarative workflow definitions (Apache Airflow, AWS Step Functions). In modern data processing pipelines or workflow orchestration systems, declarative programming is used to define workflows. Rather than writing out step-by-step procedures for task execution, you define the tasks and their dependencies, leaving the framework to manage execution order and error handling.
Example in Airflow (Python):
from airflow import DAG
from airflow.operators.dummy_operator import DummyOperator
from datetime import datetime
dag = DAG('my_dag', start_date=datetime(2021, 1, 1))
task1 = DummyOperator(task_id='start', dag=dag)
task2 = DummyOperator(task_id='end', dag=dag)
task1 >> task2 # Defining task dependency
- Configuration management (YAML, JSON). Tools like Kubernetes, Docker Compose, or CI/CD pipelines often use YAML or JSON for declarative configuration. Here, you declare the desired state of the system (e.g., which containers to run, what resources they need, environment variables) without specifying the exact sequence of actions to achieve that state.
Example in Docker Compose (YAML):
version: '3'
services:
web:
image: nginx
ports:
- "80:80"
How to Write a Declarative Code?
Writing declarative code involves focusing on describing what you want the program to do, rather than how to do it. To write declarative code, you can follow these steps:
- Identify the desired outcome. Begin by clearly defining what you want the program to achieve. This could be a data transformation, user interface display, database query, or infrastructure setup. The key is to focus on the result rather than the process.
- Use the right tools or languages. Select a language or framework that supports declarative programming. For example, SQL is used for querying databases, HTML and CSS for web design, functional programming languages like Haskell for data transformations, and frameworks like React for UI development.
- Abstract away the control flow. In declarative programming, the control flow is typically handled by the framework or system you're working with. Avoid specifying loops, conditionals, or detailed step-by-step operations. Instead, focus on expressing the desired state or operations.
- Define high-level instructions. Write statements or expressions that declare the properties or transformations you want, leaving the underlying system to figure out how to implement them. For example, instead of looping over a list to filter values, you can declare a transformation or filtering condition.
- Leverage built-in libraries or functions. Many declarative languages or frameworks provide high-level abstractions, such as database queries, UI components, or state management features, which allow you to specify what you want without getting bogged down in implementation details.
- Keep it concise and readable. One of the strengths of declarative code is its simplicity. Try to avoid unnecessary complexity by using simple and expressive syntax that communicates the desired result in a straightforward manner.
What Are the Benefits and Challenges of Using a Declarative Code?
In this section, we'll explore the benefits and challenges of using declarative code. Understanding both the strengths and limitations can help you determine when and how to effectively use declarative code in your projects.
Benefits of Declarative Code
Here are some key benefits of declarative code:
- Simpler and more readable. Declarative code tends to be more concise and easier to read since it focuses on describing what the program should do rather than how to do it. This makes it easier to understand and maintain.
- Reduced complexity. By abstracting away low-level details and control flow, declarative code simplifies complex tasks, making it easier to work with and modify.
- Less boilerplate. Declarative code often requires fewer lines and less repetitive code compared to imperative approaches. This leads to cleaner and more efficient code.
- Improved maintainability. The higher level of abstraction in declarative code means it is less tightly coupled to specific implementation details, making it easier to modify or extend in the future.
- Better focus on business logic. Developers can focus on expressing the core logic or goal of the program, rather than dealing with the underlying procedural steps, leading to more efficient problem-solving.
- Error reduction. With fewer lines of code and less manual handling of operations, declarative code reduces the chances of introducing errors, such as mismanaging loops or conditionals.
- Optimization by the framework or system. In many cases, the system (e.g., a database engine or framework) optimizes the execution of declarative code behind the scenes, leading to potentially better performance compared to manually specifying every step.
Challenges of Declarative Code
Here are some challenges associated with declarative code:
- Limited control over execution. In declarative programming, the underlying system handles how tasks are performed. This sometimes leads to inefficiencies or lack of fine-grained control over performance optimization, making it harder to tune specific parts of the process.
- Learning curve. Declarative languages and frameworks often require a different way of thinking compared to imperative ones. For developers accustomed to specifying every step in a process, transitioning to a declarative approach involves a steep learning curve.
- Debugging and tracing. Since the implementation details are abstracted away, debugging and tracing the flow of execution can be more difficult. Identifying the root cause of issues may require a deeper understanding of the framework or system managing the execution.
- Performance overheads. In some cases, declarative code introduces performance overhead due to the abstraction layers and the generalized way tasks are handled. This can be particularly problematic in performance-critical applications where fine control over resource usage is necessary.
- Less flexibility. While declarative code focuses on the what rather than the how, this sometimes limits flexibility. Customizing behavior to fit specific, complex requirements may not be as straightforward or possible within the constraints of a declarative approach.
- Abstraction misalignment. The abstractions provided by declarative languages or frameworks may not always align perfectly with the needs of the developer. In some cases, the systemโs abstraction may make it harder to express certain logic, leading to workarounds or compromises.
Declarative Code vs. Imperative Code
Letโs compare declarative code and imperative code in a table:
Aspect | Declarative Code | Imperative Code |
Definition | Describes what the program should do, abstracting away the details of how to achieve it. | Describes how the program should accomplish tasks step by step. |
Focus | Focuses on the desired outcome or result. | Focuses on the sequence of operations or steps to achieve the result. |
Control over execution | Limited control over how the task is executed. | Full control over the flow and logic of execution. |
Readability | Generally more concise and readable due to higher abstraction. | Can be harder to read, especially for complex processes. |
Complexity | Easier to maintain and extend due to simpler code structure. | Can become more complex as tasks and logic grow, requiring more management. |
Flexibility | Less flexible, as itโs harder to specify custom low-level operations. | More flexible, allowing fine-tuning and customization. |
Examples | SQL, HTML, CSS, functional programming. | C, Java, Python (with explicit loops, conditionals). |
Performance | May introduce overhead due to abstraction layers. | Can be more performant since the developer controls every step. |
Error handling | Errors can be harder to trace due to abstraction. | Easier to track errors as you have explicit control over the steps. |
Use cases | Ideal for high-level tasks like querying, UI definition, and configuration. | Best for scenarios requiring detailed control over execution flow, such as algorithms and low-level system programming. |